Integration for Jee
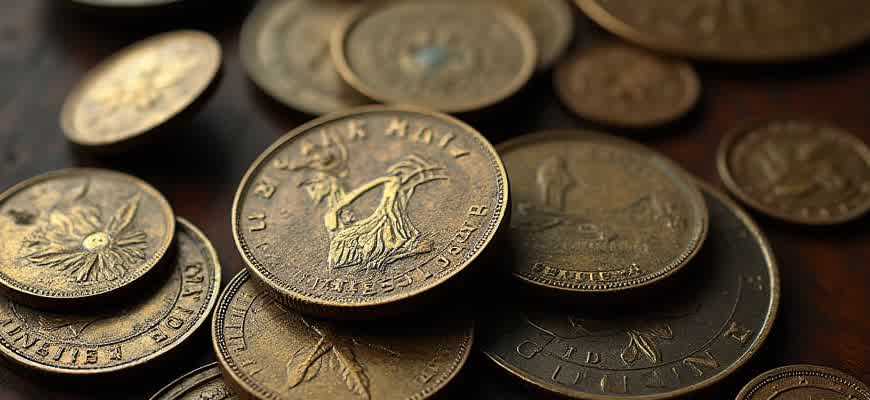
Integrating various technologies into Java EE (Enterprise Edition) environments is crucial for enhancing the capabilities of enterprise applications. By connecting external systems or components, developers can streamline business processes and ensure more efficient data handling. There are multiple approaches to integration, each suited to specific use cases and requirements.
Key Integration Methods:
- Web Services (SOAP/REST)
- Message Queues (JMS)
- Database Integration (JPA, JDBC)
- Third-Party API Connections
Important: Each integration method comes with its own set of challenges and performance considerations. It is essential to evaluate the business needs and select the most suitable method for your system architecture.
Common integration patterns include:
- Service-Oriented Architecture (SOA)
- Event-Driven Architecture
- Microservices Integration
Method | Use Case | Advantages |
---|---|---|
Web Services | Interoperability between platforms | Standardized, language-agnostic |
JMS | Asynchronous messaging | Scalable, reliable communication |
JPA | Database interaction | Object-relational mapping, simplifies persistence |
Key Considerations When Integrating Jee with Legacy Systems
When connecting Java EE (Jee) applications with legacy systems, several critical factors must be taken into account to ensure smooth integration. Legacy systems often have outdated technologies, data formats, and communication protocols, making the integration process complex. The architecture of the new system must be compatible with the older one, taking into consideration the differences in platform and language dependencies.
Moreover, the integration needs to address performance, scalability, and reliability challenges. The impact of integrating Jee applications into legacy systems could affect transaction speeds and the overall responsiveness of the system. Hence, a structured approach must be followed to mitigate potential issues and ensure a stable interface between both environments.
Challenges and Best Practices
- Data Transformation – Legacy systems may use different data structures, necessitating robust data mapping and transformation layers.
- Protocol Compatibility – Many legacy systems use proprietary or older communication protocols, requiring the implementation of adapters or middleware to bridge the gap.
- Performance Tuning – Integration can lead to slower performance; optimizations in both systems may be needed to ensure acceptable performance levels.
- Error Handling – Due to the varying degrees of reliability between legacy and modern systems, a well-defined error-handling mechanism should be put in place to ensure smooth communication.
Integration Strategy
- Assess the Existing Infrastructure: Evaluate the legacy system’s current architecture and identify potential compatibility issues.
- Define Interfaces: Design APIs and interfaces that bridge both the Jee and legacy systems.
- Testing & Validation: Implement extensive testing to validate the integrity and performance of the integration.
- Monitor and Optimize: Post-integration monitoring is crucial to identify bottlenecks or failures and make necessary optimizations.
Note: Effective integration requires careful attention to both technical and business processes to avoid disrupting ongoing operations and to ensure long-term system stability.
Summary of Key Considerations
Consideration | Impact | Solution |
---|---|---|
Data Incompatibility | Potential data loss or misinterpretation | Implement data transformation tools or middleware |
Communication Protocol Mismatch | Disrupted communication between systems | Use adapters or middleware for protocol translation |
System Performance | Decreased system speed and efficiency | Optimize both systems, use caching, or load balancing |
Error Handling | Inconsistent system behavior | Implement robust error detection and recovery processes |
Steps to Troubleshoot Common Integration Issues in Jee
Integration of Java EE (Jee) applications can often lead to various issues, ranging from communication failures to performance bottlenecks. Troubleshooting these problems requires a structured approach to identify the root causes and apply effective solutions. This guide outlines the critical steps to follow when resolving common integration problems in Jee-based environments.
Each issue in Jee integration can be linked to specific components such as messaging systems, data sources, or transaction management. Understanding the core architecture and isolating problem areas can significantly accelerate the troubleshooting process. Below are the essential steps to follow when resolving these issues.
1. Identify the Problem Area
Start by narrowing down the potential sources of failure. Common integration issues typically involve one of the following areas:
- Message queues or brokers
- Data source or database connection
- Web services or RESTful API integration
- Transaction management inconsistencies
Tip: Use logging tools to collect detailed error messages from both the client and server sides. Logs can help pinpoint exactly where the failure occurs, whether it's in the message transmission or within the database layer.
2. Verify Configuration Settings
Incorrect configuration settings are a common cause of integration issues in Jee applications. Ensure that the following configurations are correct:
- JNDI Lookups: Verify that resource lookups (e.g., data sources, JMS destinations) are correctly mapped in the application server.
- Security Credentials: Check for authentication and authorization errors related to APIs or messaging services.
- Transaction Settings: Ensure that transaction boundaries are set up correctly, especially when integrating with external systems.
Important: Misconfigured connection pools or incorrect database settings can often lead to performance degradation or failure in integration. Make sure these settings match the actual environment parameters.
3. Analyze Dependencies
Integration issues may arise due to incompatible or missing dependencies between various modules in the application. Use the following steps to verify and resolve dependency issues:
- Check for version conflicts between libraries and frameworks (e.g., JPA providers, JMS clients, etc.).
- Ensure that all required external dependencies are correctly included in the classpath.
Dependency | Version Check | Status |
---|---|---|
JPA | 2.2.0 | Correct |
JMS | 1.1.0 | Mismatch |
Note: Use dependency management tools (e.g., Maven, Gradle) to handle versioning automatically, reducing the likelihood of manual errors.
Configuring Webhooks in Jee for Real-Time Data Sync
Integrating webhooks within the Jee system can significantly improve the efficiency of data synchronization in real-time scenarios. Webhooks act as a method of pushing data to other systems or services whenever a specific event occurs, ensuring that data remains up-to-date without the need for continuous polling. By configuring webhooks, you enable an immediate data exchange, which is essential for maintaining consistency and minimizing delays.
Setting up webhooks in Jee involves a series of steps that include creating the webhook, defining events that trigger the webhook, and ensuring the proper handling of incoming data. The following steps outline the basic configuration process for establishing webhooks in Jee, enabling seamless integration with external applications.
Steps for Configuring Webhooks
- Define the Webhook Endpoint
First, create a receiving endpoint in the external application where data will be sent. This URL will be the destination for webhook notifications from Jee.
- Choose Events to Trigger the Webhook
Select specific actions or events (such as user registration, data update, or payment confirmation) in Jee that will trigger the webhook and push data to the endpoint.
- Setup Payload and Headers
Define the structure of the data payload (typically in JSON format) that Jee will send. Also, specify any necessary authentication headers to secure the webhook calls.
- Test the Webhook
Before going live, thoroughly test the webhook setup to verify that data is transmitted correctly and the receiving system processes the data as expected.
Example Webhook Configuration Table
Webhook Event | Endpoint URL | Data Payload Format |
---|---|---|
Order Created | https://example.com/webhook/order | JSON |
User Registered | https://example.com/webhook/user | JSON |
Important: Ensure that your receiving endpoint is capable of handling incoming HTTP POST requests and securely processes the webhook payload.
Optimizing Data Flow Between Jee and External Services
Efficient data exchange between Java Enterprise Edition (JEE) applications and external services is crucial for maintaining performance and reliability. Proper integration strategies not only ensure smooth communication but also help reduce latency and improve scalability. Below are key considerations and approaches to optimize this interaction.
To enhance data flow, various techniques can be employed, ranging from connection pooling to asynchronous processing. Understanding the specific needs of the system and external services is essential to determine the most appropriate integration patterns and protocols. This will lead to more efficient use of resources and reduced response times.
Key Strategies for Optimizing Data Flow
- Connection Pooling: Reduces the overhead of repeatedly establishing connections to external services, thereby speeding up data exchange.
- Asynchronous Communication: Non-blocking communication allows the application to process other tasks while waiting for external services to respond.
- Data Caching: Storing frequently requested data reduces the need to fetch it repeatedly from external services, improving performance.
- Batch Processing: Sending requests in batches minimizes the number of network calls, improving throughput.
Integration Patterns
- Request-Reply Pattern: Common for synchronous operations where the client waits for a response from the service.
- Event-Driven Pattern: Useful for systems that need to react to specific events triggered by external services.
- Publish-Subscribe Pattern: Ideal for scenarios where multiple consumers need to receive data updates from a single service.
Considerations for Efficient Data Handling
Consideration | Impact |
---|---|
Message Serialization | Efficient serialization formats, like JSON or Avro, reduce processing time and minimize payload size. |
Error Handling | Robust error handling ensures reliable recovery from failures and minimizes data loss. |
Security | Proper encryption and authentication mechanisms protect sensitive data during transmission. |
Note: Optimization is a continuous process, requiring monitoring and adjustments based on evolving system requirements and external service capabilities.
How to Secure Data Transfers in Jee Integration
When integrating various systems in the Java Enterprise Edition (JEE) environment, securing data transfers is paramount to ensure the confidentiality, integrity, and authenticity of the transmitted information. This becomes especially critical when sensitive data such as personal details, payment information, or corporate secrets are involved. Effective security practices must be implemented to protect data in transit from potential cyber threats, data breaches, or unauthorized access.
One of the key components in securing data transfers in JEE integration is the proper configuration of encryption protocols, secure communication channels, and authentication mechanisms. These methods help in ensuring that the data being transferred between systems is protected and only accessible to authorized parties.
Steps to Secure Data Transfers
- Use HTTPS for Communication – Ensure that all data exchanged between systems is encrypted using HTTPS (Hypertext Transfer Protocol Secure). This provides encryption through SSL/TLS protocols, ensuring that data is not intercepted or altered during transmission.
- Implement Authentication and Authorization – Use authentication methods like OAuth, JWT (JSON Web Tokens), or basic authentication to ensure that only authorized users or systems can access sensitive data.
- Leverage Secure SOAP or RESTful Web Services – For service-based integrations, SOAP and RESTful services must be configured to support security standards such as WS-Security or OAuth to provide message-level security and ensure that only validated and authorized messages are processed.
- Encrypt Sensitive Data – Any sensitive data that must be sent over the network should be encrypted using strong encryption algorithms such as AES (Advanced Encryption Standard) to prevent unauthorized decryption.
Important Security Considerations
It is essential to keep the software and libraries used for encryption up-to-date, as vulnerabilities can often be discovered in older versions.
- Ensure that SSL/TLS certificates are valid and properly configured.
- Use mutual authentication for added security between services.
- Consider using IP whitelisting to restrict access to trusted systems only.
- Monitor and log data transfer activities to detect any suspicious behavior.
Key Tools for Securing Data Transfers
Tool | Purpose | Recommendation |
---|---|---|
SSL/TLS | Encrypts communication over the network | Always use strong cipher suites and keep certificates updated. |
OAuth | Secures access to APIs and services | Implement token-based authentication for API security. |
JWT | Provides a secure way to transfer user information | Use for stateless authentication in distributed systems. |
Best Practices for Testing JEE Integrations
When working with Java EE (JEE) integrations, thorough testing is crucial to ensure the seamless interaction between components. Given the complexity of enterprise systems, it’s essential to apply structured approaches for validating integrations. This helps in identifying issues early in the development lifecycle, reducing costs, and improving system stability. The testing approach should cover both functional and non-functional requirements to ensure the integration meets all expected performance and security standards.
Effective integration testing involves a range of methodologies and tools tailored to different aspects of JEE applications. These include testing data exchanges between services, verifying communication protocols, and evaluating error handling mechanisms. Adopting best practices ensures a reliable and maintainable system, minimizing downtime and operational risks.
Key Testing Practices for JEE Integrations
- Unit Testing of Integration Components: Focus on testing individual integration points, such as EJBs or web services, to ensure they function as expected in isolation.
- Mock External Services: Use mock objects or frameworks like WireMock to simulate external dependencies, ensuring that integration tests do not rely on actual external systems.
- Automated Regression Testing: Set up automated tests that can be executed after each integration change to verify that no new issues have been introduced.
- End-to-End Testing: Conduct tests that simulate actual user interactions and ensure the integration works in a real-world scenario.
- Load and Stress Testing: Evaluate how the integration handles high traffic and heavy data loads to identify potential performance bottlenecks.
Common Tools for Testing JEE Integrations
Tool | Description |
---|---|
JUnit | Widely used framework for unit testing Java applications, including integration components. |
Arquillian | A testing platform designed for Java EE applications, enabling integration tests within a container environment. |
Mockito | Framework for mocking objects, useful for simulating external service calls during integration testing. |
SoapUI | Tool for testing web services and SOAP-based integrations in JEE environments. |
Note: Proper exception handling and transaction management are key areas to test when working with JEE integrations. Make sure that failures in one component do not lead to cascading failures throughout the system.
Scaling Your Jee Integration as Business Demands Grow
As businesses expand, the need for robust integration solutions becomes increasingly critical. Your Jee system must evolve to accommodate the growing demands of your organization while maintaining optimal performance. Whether it's handling larger datasets, more complex workflows, or additional user requirements, scaling your integration infrastructure is key to sustaining business operations smoothly and efficiently.
Implementing scalable solutions ensures that your Jee integration can handle increasing workloads without compromising system stability or performance. This process involves optimizing your integration architecture, adopting cloud-based solutions, and continuously monitoring system metrics to anticipate future needs. In this context, the ability to scale efficiently is a competitive advantage that enhances both the speed and reliability of business processes.
Key Strategies for Scaling Jee Integration
- Modular Architecture: Break down your integration components into smaller, manageable modules. This enables flexible scaling and easier updates to individual elements as business needs change.
- Cloud-Based Solutions: Leverage cloud infrastructure to dynamically scale resources based on real-time demand. This reduces costs and ensures high availability.
- Load Balancing: Implement load balancing techniques to distribute traffic evenly across servers, preventing bottlenecks and ensuring system performance during peak usage periods.
- Automation: Automate repetitive tasks and workflows to improve efficiency and reduce manual intervention as the scale of operations increases.
Scalable integration ensures that your system remains resilient under growing demand, allowing you to maintain consistent service delivery as your business expands.
Considerations for Future Growth
As your business scales, it's essential to assess potential integration challenges early on. Monitoring system performance and conducting regular audits of integration workflows will help identify inefficiencies. Below is a table outlining key considerations for scaling Jee integration effectively:
Consideration | Action |
---|---|
Data Volume | Increase storage capacity and optimize database queries for faster processing. |
System Latency | Optimize API calls and implement caching strategies to reduce response times. |
User Access | Scale user authentication and authorization systems to handle increased traffic. |