R Generate Sequence of Numbers
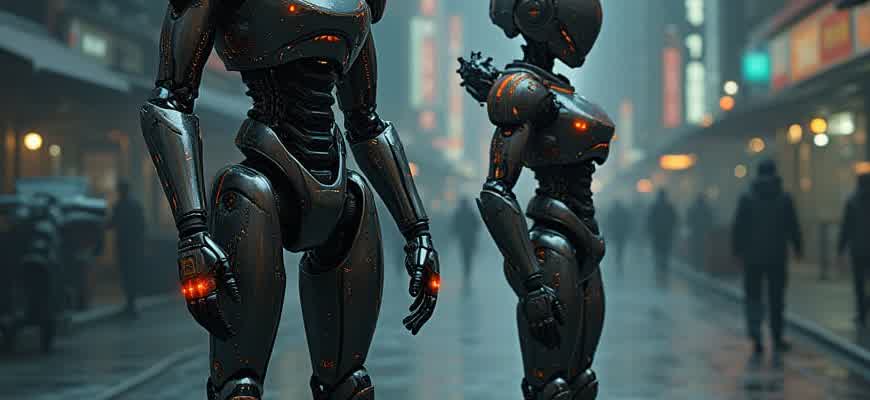
In R, generating a sequence of numbers is a fundamental task often needed in data analysis, modeling, or simulation. R provides several ways to create sequences, from simple ranges to more complex patterns. These methods are integral for iterative processes and data manipulation.
One of the most commonly used functions for this purpose is seq(), which allows you to generate a sequence of numbers with a defined step size and range. It can be customized with a variety of parameters to suit specific needs.
- seq(from, to, by): Generates a sequence from a specified starting point to an endpoint, with a defined step size.
- seq(from, to, length.out): Creates a sequence with a defined length, dividing the range evenly.
For example, the code seq(1, 10, by = 2) will generate the sequence: 1, 3, 5, 7, 9.
Another useful function is rep(), which repeats elements in a sequence either a specified number of times or until a target length is reached.
- rep(x, times): Repeats the elements of x a fixed number of times.
- rep(x, length.out): Repeats the elements to match the target length.
Function | Description |
---|---|
seq() | Generates a sequence of numbers within a defined range. |
rep() | Repeats elements of a vector to create a desired pattern. |
Generating Numerical Sequences in R for Your Data Projects
R provides multiple functions that allow you to easily generate numerical sequences, which are essential when working with large datasets or performing simulations. Sequences can be useful for creating time series, running iterative models, or simply filling vectors with specific patterns of numbers. Below, we will explore the most common methods used in R to generate sequences of numbers for various data processing tasks.
One of the primary functions for generating sequences in R is the seq() function. This versatile tool enables the user to specify start, end, and step sizes, which gives full control over the generated sequence. Additionally, the seq.int() function allows for even more optimized and quicker sequence creation in certain situations. Understanding how to leverage these functions will significantly improve the efficiency of your data-related workflows.
Key Functions for Sequence Generation in R
- seq() - Generates a sequence of numbers based on defined parameters such as start, end, and step size.
- seq.int() - Similar to seq(), but optimized for integer sequences.
- rep() - Repeats a specified value or sequence multiple times.
To generate sequences in R, you simply need to define a few parameters. Here is an example using seq() to create a sequence from 1 to 10 with a step size of 2:
seq(1, 10, by = 2)
Additionally, seq_len() and seq_along() are often used to generate sequences when dealing with indexed data, such as looping through the length of vectors or data frames.
Important: Always consider whether you need an inclusive or exclusive range when generating sequences, as this can impact your analysis, especially in time series or iterative models.
Example Sequence Generation in R
- Use seq() to generate a sequence from 0 to 100 with a step size of 5:
seq(0, 100, by = 5)
- Generate a sequence of length 10 from 1 to 20 using length.out:
seq(1, 20, length.out = 10)
- Repeat a number 5 times with rep():
rep(1, times = 5)
Function | Example | Output |
---|---|---|
seq() | seq(1, 10, by = 2) | 1 3 5 7 9 |
seq_len() | seq_len(5) | 1 2 3 4 5 |
rep() | rep(3, times = 4) | 3 3 3 3 |
Tip: Use the length.out parameter for creating evenly distributed sequences with a fixed number of elements, useful for simulations or graph plotting.
Creating a Simple Numeric Sequence in R with seq()
In R, generating a series of numbers is a common task that can be easily accomplished using the seq() function. This function allows you to create sequences with specified intervals, making it highly versatile for various programming needs. Whether you're interested in generating a range of numbers from one value to another or creating more complex numeric patterns, seq() is the go-to tool.
The basic syntax of the seq() function is simple: seq(from, to, by). Here, the "from" argument specifies the starting point, the "to" argument defines the end point, and the "by" argument sets the step size between numbers. If you omit the "by" parameter, R will automatically choose an appropriate step size.
Basic Usage of seq()
- Basic sequence with step size: Generate numbers from 1 to 10 with a step size of 2:
seq(1, 10, by = 2)
- Sequence with specified length: Generate 5 numbers between 1 and 10:
seq(1, 10, length.out = 5)
Note that when the "length.out" argument is used, R calculates the step size automatically to fit the specified number of elements.
Example: Creating Sequences
Here is a simple table of examples to help visualize the different ways to use seq():
Command | Result |
---|---|
seq(1, 5, by = 1) |
1 2 3 4 5 |
seq(0, 1, length.out = 6) |
0 0.2 0.4 0.6 0.8 1 |
As shown in the examples above, the seq() function can be tailored to meet your specific requirements, making it a powerful tool for numerical sequence generation in R.
Generating Number Sequences with Custom Steps in R
In R, generating number sequences with a defined increment is a common task, often required in data analysis, simulations, or when creating custom intervals. This process allows you to control both the starting and ending values of the sequence, as well as the step size between consecutive numbers. One of the most common ways to create such sequences is by using the seq() function, which is highly flexible for different types of ranges.
The seq() function can generate sequences with a custom step by specifying the by argument. This argument defines the interval between numbers in the sequence. Additionally, you can control the sequence's length using the length.out or to argument, depending on your needs. Below, we will explore some basic examples of creating sequences with varying increments.
Creating Sequences with Custom Increments
- Using seq(): A straightforward method to generate a sequence by setting the start, end, and step size.
- With for loops: Generating sequences programmatically in custom ways when more control is needed.
- Using the colon operator: A compact method for creating sequences with a step of 1 (or any other integer) between the start and end points.
Here's an example of creating sequences using the seq() function:
seq(from = 1, to = 10, by = 2) # Creates sequence: 1, 3, 5, 7, 9
The seq() function allows for easy creation of sequences with varying increments, and the by parameter is used to define the step size.
Additionally, it is possible to generate sequences with specific step sizes in a variety of formats:
Method | Example | Output |
---|---|---|
Using seq() | seq(1, 10, by = 3) | 1, 4, 7, 10 |
Using colon operator | 1:5 | 1, 2, 3, 4, 5 |
Using a for loop | for(i in seq(1, 10, by = 2)) { print(i) } | 1, 3, 5, 7, 9 |
In summary, creating custom number sequences in R is highly flexible. The seq() function, in particular, is a powerful tool that enables users to define sequences with any desired increment, providing significant control over the sequence's creation process.
How to Set Custom Start and End Points for Your Number Sequences in R
When generating sequences in R, the flexibility to adjust the starting and ending points is crucial for tailoring sequences to specific needs. By manipulating the parameters of the sequence-generating functions, you can control where the sequence begins and ends. This allows you to work with custom ranges of numbers for tasks like data analysis, simulations, or algorithm testing.
R provides several functions that allow for easy customization of sequence ranges. These functions can be adjusted to produce sequences with any start and end values, as well as specific step increments. Below are the key methods to modify the start and end points of a sequence in R.
Customizing Sequence Start and End Points Using `seq()`
The `seq()` function in R is one of the most flexible tools for creating numeric sequences. By adjusting its parameters, you can easily control both the starting and ending points of the sequence.
- Start Point: The first number in the sequence is defined by the `from` argument.
- End Point: The last number in the sequence is controlled by the `to` argument.
- Step Size: The increment between numbers is set by the `by` argument, which allows fine control over the spacing of the numbers.
Important Note: If the `by` argument is left unspecified, R will automatically calculate an appropriate step size based on the total number of points in the sequence.
Example of Sequence Customization
- Creating a sequence from 5 to 50 with a step size of 5:
seq(from = 5, to = 50, by = 5)
This will generate the following sequence: 5, 10, 15, 20, 25, 30, 35, 40, 45, 50.
Advanced Customization Using `length.out`
Sometimes, you may want to define the total number of elements in the sequence rather than specifying the step size. This is where the `length.out` parameter comes into play.
Important Note: The `length.out` argument specifies how many numbers to generate between the `from` and `to` values. R will automatically adjust the step size to evenly distribute the numbers within the given range.
- If you want a sequence with 10 numbers between 1 and 100, use:
seq(from = 1, to = 100, length.out = 10)
This will generate a sequence with 10 evenly spaced numbers between 1 and 100.
Summary of Parameters
Parameter | Description |
---|---|
from | Starting value of the sequence. |
to | Ending value of the sequence. |
by | Step size or interval between numbers. |
length.out | Number of elements to generate in the sequence. |
Generating Random Sequences for Simulation Purposes in R
In many simulation scenarios, it is essential to generate sequences of random numbers to mimic real-world processes or to model stochastic systems. R provides a wide range of functions for creating random sequences, each with varying properties to suit different types of simulations. For example, random number sequences can be used in Monte Carlo simulations, risk analysis, or in simulations of natural phenomena such as weather or stock prices. These sequences are typically generated from specific distributions like uniform, normal, or binomial, depending on the requirements of the model.
R’s random number generation capabilities are highly flexible, offering several functions that can be used for generating sequences of random numbers. Some of the most commonly used functions include runif() for uniformly distributed numbers, rnorm() for normal distributions, and rbinom() for binomially distributed numbers. These functions allow users to generate random values for simulations, and they can also specify the sample size, mean, standard deviation, or other parameters for the distribution being used.
Generating Random Sequences
To generate random sequences in R, one can use the following functions depending on the desired distribution:
- runif(n, min, max): Generates n random numbers from a uniform distribution between min and max.
- rnorm(n, mean, sd): Generates n random numbers from a normal distribution with a specified mean and standard deviation.
- rbinom(n, size, prob): Generates n random numbers from a binomial distribution, with a given size and probability.
Example: Generating a Random Sequence for a Simulation
- Set the seed for reproducibility:
set.seed(123)
. - Generate 100 random numbers from a normal distribution with a mean of 50 and a standard deviation of 10:
random_sequence <- rnorm(100, mean = 50, sd = 10)
. - Inspect the first few values:
head(random_sequence)
.
The generated numbers can now be used in further analysis, such as simulating scenarios or testing statistical models.
It is important to note that random number generation in R is pseudo-random, meaning it is generated using an algorithm. For truly random values, hardware-based random number generators or external libraries might be needed.
Random Numbers for Simulation Models
Using random sequences for simulation models allows you to explore different outcomes by mimicking uncertainty or variability. For example, in a financial simulation, a sequence of random stock price changes can be generated using a random walk model. This allows analysts to estimate the probability of different financial outcomes based on historical data.
Function | Distribution Type | Parameters |
---|---|---|
runif() | Uniform | n, min, max |
rnorm() | Normal | n, mean, sd |
rbinom() | Binomial | n, size, prob |
Using the seq_along() Function to Create Sequences from Vectors
In R, one common task is to generate sequences based on the length of an existing vector. The seq_along() function is designed to simplify this task by producing a sequence from 1 to the length of a given vector. This function can be useful when you need to iterate over the elements of a vector or apply specific transformations while maintaining the original structure of the data.
The seq_along() function works by automatically determining the number of elements in a vector and creating a sequence of integers from 1 to that number. This is especially useful when working with larger datasets or dynamically sized vectors, where manually specifying the length of the sequence would be inefficient and error-prone.
Example of seq_along() in Action
- Given a vector vec with elements:
vec <- c(3, 5, 7, 9)
, usingseq_along(vec)
will return the sequence:1, 2, 3, 4
. - When iterating over elements of vec, the sequence generated helps to track the index of each element.
Note: The length of the sequence produced by seq_along() will always match the number of elements in the vector. This makes it especially useful when performing element-wise operations or accessing specific indices.
Practical Application
- In data manipulation tasks, you might want to apply a function to each element in a vector. By using
seq_along()
, you can easily iterate over the indices and apply transformations based on the index. - For example, if you're working with a vector of numbers and you want to add a unique value to each element based on its position,
seq_along()
allows you to reference the correct index for such operations.
Comparison Table
Vector | Result of seq_along() |
---|---|
c(2, 4, 6) | 1, 2, 3 |
c("apple", "banana", "cherry", "date") | 1, 2, 3, 4 |
Efficient Techniques for Generating Large Number Sequences in R
Creating large sequences in R efficiently is crucial when working with extensive datasets or simulations. Whether you're generating sequences of integers, floats, or custom patterns, R provides several methods that balance speed and memory usage. In many scenarios, inefficient sequence generation can lead to excessive computation time or memory issues. Optimizing these processes ensures smooth performance, especially when handling large datasets in statistical analysis or machine learning tasks.
R offers various ways to generate sequences, but it's important to choose the right method depending on the context. Some functions are specifically designed to minimize memory usage, while others focus on computational speed. Understanding these techniques can help developers avoid unnecessary resource consumption, especially when sequences involve millions of elements.
Methods for Efficient Sequence Creation
Here are a few techniques commonly used in R to create large sequences efficiently:
- seq(): The
seq()
function allows users to generate sequences with specific intervals. It is flexible and can be used for both numeric and integer sequences. - seq_len() and seq_along(): These are optimized functions for generating sequences of integer values.
seq_len()
is particularly memory-efficient, especially when the sequence length is known in advance. - rep(): This function repeats a set of values. It is highly efficient for generating repeated patterns within sequences.
Best Practices for Working with Large Sequences
To create sequences with minimal memory overhead, it’s recommended to use the following strategies:
- Pre-allocate vectors: Instead of dynamically expanding a vector, allocate memory upfront to prevent costly resizing during sequence creation.
- Vectorization: Use vectorized operations to avoid loops. R is optimized for vectorized operations, which is significantly faster than iterative methods.
- Avoid unnecessary copies: When generating sequences, avoid creating unnecessary copies of data, especially when the sequence elements can be directly manipulated in-place.
Performance Comparison
Method | Memory Efficiency | Speed |
---|---|---|
seq() | Good | Moderate |
seq_len() | Excellent | Fast |
rep() | Good | Fast |
Always choose the simplest method that meets your needs. For very large sequences, the
seq_len()
function is often the best choice in terms of memory and computational efficiency.
Handling Non-Uniform Sequences with Custom Step Sizes in R
In R, generating sequences with a non-uniform step size requires a more tailored approach compared to simple sequences. When working with irregular steps between numbers, the standard sequence functions such as `seq()` might not suffice. Instead, it is necessary to define a custom sequence generation method or adjust the step values manually to achieve the desired result. This allows greater flexibility when dealing with data that requires unequal intervals between elements.
For sequences where the difference between consecutive numbers is not constant, the `seq()` function can still be used, but you must provide a custom vector of step values. Alternatively, one can use loops or functions like `sapply()` to construct a sequence manually. By applying these methods, users can efficiently manage sequences where the steps are not evenly distributed.
Creating Custom Sequences with Variable Steps
One approach to generating sequences with custom intervals involves explicitly defining the steps in a vector. Here is how to generate a sequence with different intervals between numbers:
- Define the starting value.
- List the step sizes for each transition between numbers.
- Use a loop or vectorized operations to create the sequence.
Note: For highly irregular sequences, using loops with predefined step sizes is often the most straightforward approach.
Example of Creating Custom Step Sequences
The following example demonstrates how to generate a non-uniform sequence with varying step sizes in R:
start_value <- 1 step_sizes <- c(2, 5, 3, 7) sequence <- numeric(length = length(step_sizes) + 1) sequence[1] <- start_value for(i in 2:length(sequence)) { sequence[i] <- sequence[i - 1] + step_sizes[i - 1] } sequence
This script will produce the sequence: 1, 3, 8, 11, 18
Table of Custom Sequence Examples
Start Value | Step Sizes | Generated Sequence |
---|---|---|
1 | 2, 5, 3, 7 | 1, 3, 8, 11, 18 |
0 | 4, 6, 2 | 0, 4, 10, 12 |
Automating Sequence Generation with Loops in R
Loops in R can be a powerful tool for automating repetitive tasks, such as generating sequences of numbers. By using loops, you can create more complex sequences without manually entering each value. This approach is efficient when dealing with large datasets or when the sequence needs to be generated dynamically based on specific parameters.
There are several types of loops in R, with the most commonly used being the for loop. This loop allows you to iterate through a set of numbers or values, performing the same operation at each step. By incorporating loops, you can automate the generation of sequences for various tasks such as simulations, data analysis, and testing different algorithms.
Using Loops to Generate Sequences
When generating a sequence using a loop, the for statement provides a straightforward way to control the flow and increment values. Below is an example of how to use a loop to create a sequence of numbers from 1 to 10:
for (i in 1:10) { print(i) }
This loop will iterate through the numbers 1 to 10, printing each value in the console. You can modify this loop to generate more complex sequences by adjusting the range or applying mathematical operations inside the loop body.
Steps for Sequence Generation
- Define the range or limits of your sequence.
- Set the loop to iterate through each value in the specified range.
- Apply any necessary operations (e.g., addition, multiplication) inside the loop.
- Store or display the results at each step.
Advanced Sequence Generation
For more customized sequence generation, you may need to combine loops with conditional statements or other functions. For instance, you can generate a sequence where the step size is dynamic, or you can apply mathematical operations to modify the numbers as they are generated.
"Loops offer flexibility and efficiency in generating sequences that would otherwise require manual input or complex functions."
Here’s an example of generating a sequence where each number is doubled at every iteration:
sequence <- numeric(10) for (i in 1:10) { sequence[i] <- 2^i } print(sequence)
This code creates a sequence where each element is a power of 2, starting from 21 to 210.
Benefits of Using Loops
- Efficiency: Loops eliminate the need for manual input, saving time when generating large sequences.
- Flexibility: You can easily adjust the loop parameters to change the sequence's characteristics.
- Customization: Loops can be combined with other functions and conditional statements for advanced sequence generation.
Summary Table
Type of Loop | Use Case |
---|---|
For Loop | Generate a sequence over a defined range |
While Loop | Generate a sequence based on a condition |