Integration in Reflex
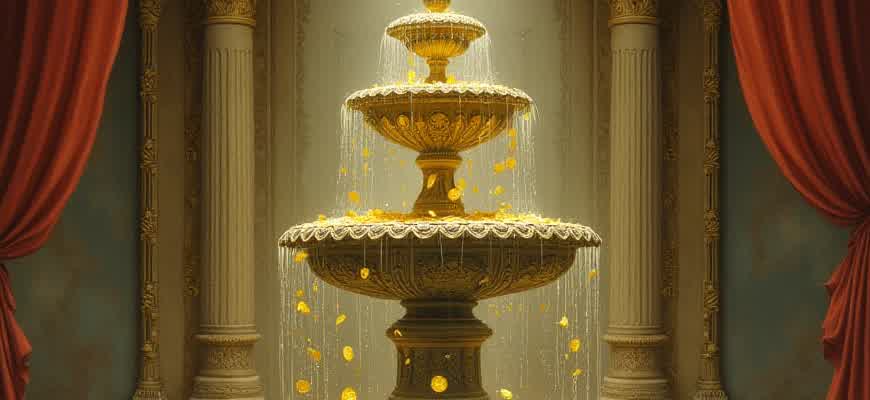
In the context of Reflex, embedding additional logic or behavior into the reactive system requires precise coordination between event streams and dynamic computations. This is achieved through mechanisms that allow separate components to interact within the same reactive timeline. One such mechanism is the composition of event-producing functions using the MonadWidget or PerformEvent interfaces.
Proper integration relies on maintaining consistency across dynamic updates and event propagation chains.
There are several concrete approaches to embedding and managing reactive components:
- Using Dynamic types to track time-varying values across user inputs and backend changes.
- Leveraging Event combinators to synchronize asynchronous triggers and feedback loops.
- Combining widgets using runWithReplace to allow conditional logic based on runtime behavior.
Step-by-step integration flow:
- Define the required reactive inputs using
textInput
,button
, or similar primitives. - Wrap reactive logic using
performEvent
for asynchronous effects (e.g., API calls). - Manage layout or visibility using
dyn
orwidgetHold
.
Function | Description |
---|---|
holdDyn |
Captures the latest value from an event stream into a dynamic container. |
switchHold |
Dynamically replaces one event stream with another at runtime. |
performEvent_ |
Executes side effects on event firing without returning a value. |
Configuring Initial API Links in Reflex
Before implementing external data exchange in a Reflex application, it's essential to configure the foundational API access points. This involves defining the base URLs, authentication protocols, and expected response formats. These settings ensure stable communication between Reflex and third-party services.
The configuration process typically starts in the backend logic layer, where API endpoints are declared. Integration parameters such as headers, tokens, and request limits must be included explicitly to avoid runtime errors and maintain compliance with the API provider's specifications.
Steps to Establish API Communication
- Identify and document the external service endpoints.
- Generate or obtain necessary credentials (API keys, OAuth tokens).
- Define connection parameters in Reflex backend logic using `reflex.constants` or environment variables.
- Test request/response cycles using Reflex async handlers.
Note: All credentials should be stored securely using environment variables or a secret management system. Avoid hardcoding sensitive data into your Reflex scripts.
- Use `httpx.AsyncClient` for non-blocking API requests.
- Handle exceptions explicitly to prevent application crashes.
- Parse and validate incoming data formats (e.g., JSON, XML) before rendering in UI.
Component | Description | Required |
---|---|---|
Base URL | Root path to the API provider | Yes |
Authorization Header | Contains tokens or API keys | Yes |
Timeout Settings | Limits request duration | No |
Secure Handling of Access Credentials for External APIs
When connecting with external platforms such as payment gateways, analytics tools, or cloud services, managing authentication credentials becomes a critical aspect of system security. These credentials, often in the form of bearer tokens or API keys, require secure storage, lifecycle control, and controlled access within your infrastructure.
Effective integration requires not only storing the tokens securely but also ensuring they are refreshed when expired and rotated regularly to prevent misuse. Integration layers should be responsible for isolating token logic, allowing the rest of the application to operate without direct exposure to sensitive data.
Best Practices for Token Lifecycle Management
- Store tokens in encrypted storage (e.g., HashiCorp Vault, AWS Secrets Manager).
- Use short-lived tokens where possible to reduce risk in case of leaks.
- Implement automated token renewal using refresh tokens or service credentials.
Note: Never expose tokens in client-side code or logs. Always ensure server-side control and audit logging.
- Validate token scopes and permissions on each request to enforce least privilege.
- Monitor usage patterns and revoke compromised credentials immediately.
- Rotate long-term credentials regularly and on employee offboarding.
Service | Token Type | Rotation Method |
---|---|---|
Google Cloud | OAuth2 Access Token | Auto-refresh via Refresh Token |
Stripe | Secret Key | Manual rotation via Dashboard/API |
GitHub | Personal Access Token | Time-limited; manual renewal |
Adapting External Data Models to Reflex Structure
When incorporating external datasets into a Reflex-based system, a direct one-to-one mapping is rarely feasible. The incoming structure may include nested hierarchies, non-standard field names, or data types not natively supported by Reflex. To align this data with the Reflex schema, transformation logic must be introduced during the ingestion or pre-processing phase.
This transformation often involves flattening nested elements, renaming fields to match Reflex conventions, and converting data types. For instance, timestamps in Unix format may need to be converted to ISO 8601 strings. The goal is to produce a clean, semantically compatible data layer that Reflex can process without ambiguity.
Common Transformation Steps
- Normalize field names to match Reflex naming patterns.
- Convert data types (e.g., stringified numbers to integers).
- Flatten deeply nested structures for simplified access.
- Validate mandatory fields are present and populated.
- Inspect external schema and document field types.
- Define transformation rules for each field group.
- Implement mapping functions using Reflex's pre-processor or ETL pipeline.
- Test transformed output against target Reflex schema definitions.
Note: Always validate transformed data using Reflex's schema enforcement tools before committing changes to production pipelines.
External Field | Transformed Field | Conversion Logic |
---|---|---|
userId | user_id | Renamed to match snake_case convention |
createdAt | created_at | Timestamp converted from epoch to ISO 8601 |
address.city.name | city_name | Flattened nested structure |
Automating Processes Using External Webhook Signals
When a third-party service sends data to a specific URL, it can act as a remote signal to trigger internal processes. These signals, known as webhooks, allow systems to respond in real time to events such as form submissions, user signups, or payment confirmations.
Inside Reflex, such signals can be captured and processed instantly, launching predefined operations without human intervention. This enables seamless integration with external platforms like Stripe, GitHub, or custom CRMs.
Webhook-Driven Execution Flow
Reflex listens for HTTP requests on exposed endpoints and maps the payload to internal workflow parameters.
- Define an endpoint to accept incoming data
- Validate payload format and source authenticity
- Pass structured data to a corresponding logic block
- Create a webhook listener in Reflex with the desired URL
- Bind the listener to a specific workflow action
- Test the connection using a known payload
External Event | Trigger Action | Workflow Result |
---|---|---|
Payment completed (Stripe) | Parse payload and verify signature | Send receipt, update order status |
Issue opened (GitHub) | Extract issue metadata | Notify dev team, create task |
Error Management in Reflex Integrations
When Reflex interacts with third-party systems, improper or unexpected responses can disrupt the workflow. To ensure reliability, the system must identify, categorize, and act on these error signals in real-time.
Error handling involves structured parsing of response payloads, logging critical details, and executing fallback logic where applicable. Each platform may return errors differently, making standardized interpretation essential.
Key Mechanisms for Response Validation
- Response Code Evaluation: HTTP status codes are assessed first. Any code outside the 2xx range triggers the error handling layer.
- Payload Inspection: Even 2xx responses may contain logical errors in the body; keys like error_code or message are analyzed.
- Timeout Detection: If no response is received within a set interval, the request is marked as failed and retried based on retry policy.
Robust integrations treat every response as a potential failure until fully validated.
- Classify error type: network, platform-specific, authentication, or logical failure.
- Record all details in centralized logs with correlation IDs.
- Notify relevant systems or users if thresholds are exceeded.
Error Type | Source | Example |
---|---|---|
Transport Error | Network Layer | Connection timeout |
Authentication Error | API Gateway | 401 Unauthorized |
Logical Failure | Application Layer | Invalid order ID in payload |
Monitoring Integration Logs and Debugging Failures
Effective monitoring of integration processes is crucial to ensuring smooth data exchange and system interaction. Logs play a vital role in tracking the flow of information between different systems, allowing you to quickly detect issues and take necessary actions. When integration failures occur, identifying the root cause becomes paramount, and logs are essential for this purpose. Systematic logging and error monitoring provide detailed insights into each step of the integration process, making it easier to identify where something went wrong.
To successfully debug integration failures, it is necessary to analyze logs effectively. They often contain a variety of information, including error messages, timestamps, and specific system responses. By carefully reviewing these logs, you can uncover patterns or pinpoint exact issues that need to be addressed. Utilizing the right tools and strategies for log monitoring can drastically reduce downtime and prevent future failures.
Steps to Monitor Integration Logs
- Set up centralized logging to capture all relevant data from integration points.
- Ensure proper log levels are defined (e.g., ERROR, WARN, INFO) to filter data efficiently.
- Implement automated alerts for critical errors to trigger immediate responses.
- Regularly review logs to identify recurring issues and prevent future failures.
Common Causes of Integration Failures
- Network issues: Connectivity problems can disrupt data transmission between systems.
- Data format mismatches: Inconsistent data structures or invalid input data can cause failures.
- Timeouts: Systems not responding in time may result in failed transactions.
Tip: Always ensure that integration services are regularly updated and well-documented to avoid common failures.
Helpful Debugging Strategies
Issue | Possible Solution |
---|---|
Failed API request | Check the request parameters and verify API endpoint availability. |
Incorrect data format | Validate data input before transmitting and ensure compatibility with target system. |
Slow response time | Increase system resources or optimize queries to reduce response time. |
Scaling Reflex Integrations for High Data Volumes
Handling large volumes of data in Reflex integrations requires careful consideration of performance, scalability, and reliability. When processing massive datasets, both the architecture and design of integrations need to be optimized to prevent bottlenecks and ensure seamless operation. Efficient management of high-frequency data influxes is crucial for maintaining response times and system integrity under load.
One of the key challenges when scaling Reflex integrations is ensuring that the underlying infrastructure can handle the processing demands. Leveraging cloud-based solutions, distributed computing, and efficient data storage mechanisms can help achieve optimal performance at scale. It's also essential to implement real-time processing and batch processing strategies to balance speed and data throughput.
Optimization Strategies for Large-Scale Integrations
- Load Balancing: Distribute data processing tasks evenly across multiple servers or instances to prevent overloading any single resource.
- Parallel Processing: Utilize multi-threading and parallel computing to process large data volumes simultaneously, reducing overall processing time.
- Efficient Data Storage: Use distributed file systems and databases designed for high-volume data, such as NoSQL solutions, to handle large-scale data storage.
- Data Caching: Implement caching mechanisms to store frequently accessed data, reducing the need for repeated database queries and improving speed.
Key Considerations for High-Volume Data Integrations
- Latency Management: Minimize latency by optimizing data flow and choosing low-latency network connections.
- Data Integrity: Ensure data accuracy and consistency across all systems, especially during heavy load periods.
- Monitoring and Alerts: Set up real-time monitoring tools and alerting systems to identify potential issues before they impact system performance.
Important: Scaling Reflex integrations efficiently requires an iterative approach to continuously monitor and adjust the system for optimal performance under varying data loads.
Technical Solutions for Scaling
Solution | Benefits |
---|---|
Distributed Cloud Infrastructure | Scalable resource allocation, high availability, and fault tolerance. |
Data Sharding | Improved data distribution, better load handling, and reduced risk of database overload. |
Asynchronous Data Processing | Improved responsiveness by decoupling data processing from real-time requirements. |