R Sentiment Analysis Example
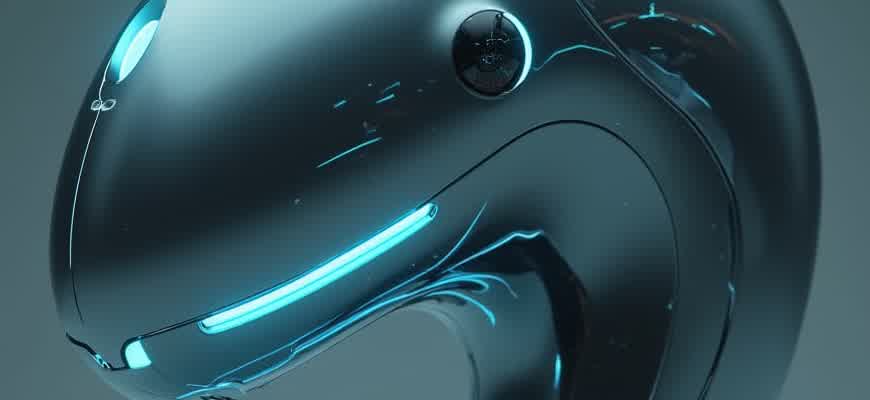
Sentiment analysis is a powerful technique used to understand and extract subjective information from textual data. In the R programming language, there are several tools and libraries that make it easy to perform sentiment analysis on various types of data. By evaluating the tone, emotions, and opinions expressed in text, sentiment analysis can provide valuable insights, whether it's for customer feedback, social media posts, or product reviews.
Steps for Conducting Sentiment Analysis in R:
- Data Collection: Gather the text data you want to analyze.
- Text Preprocessing: Clean the data by removing unwanted characters and stop words.
- Sentiment Scoring: Use a sentiment dictionary or machine learning model to assign scores to the text.
- Data Visualization: Plot the sentiment scores to understand trends and patterns.
For example, using the tidytext package in R, you can analyze the sentiment of a series of product reviews and determine if they are mostly positive or negative. Below is an example of how sentiment data might be presented:
Review | Sentiment Score |
---|---|
Great product, very useful! | Positive |
Not as expected, very disappointing. | Negative |
Average quality, could be better. | Neutral |
"Sentiment analysis can be a game-changer for businesses looking to gain real-time feedback from customers or the public."
How to Install R and Required Packages for Sentiment Analysis
Setting up R for sentiment analysis is a straightforward process, requiring the installation of both the R programming language and several key packages. In this guide, we will walk through the installation steps for R and the necessary libraries, which are crucial for performing sentiment analysis tasks effectively.
R is an open-source statistical programming language, commonly used for data analysis and visualization. Once R is installed, you will need to install a few specific packages that will allow you to process text data and analyze sentiment. Below are the steps to get started.
Step-by-Step Installation
- Download and install R from the official website: CRAN
- Open RStudio (a popular IDE for R) or the R console.
- To install the necessary sentiment analysis packages, use the following commands:
install.packages("tidyverse") install.packages("textdata") install.packages("syuzhet") install.packages("tm")
Once installed, you can load the packages into your session by running:
library(tidyverse) library(textdata) library(syuzhet) library(tm)
Required Packages Overview
Here is a brief overview of the packages mentioned above:
Package Name | Purpose |
---|---|
tidyverse | Provides a collection of R packages for data manipulation and visualization. |
textdata | Allows easy access to datasets for text mining, including sentiment lexicons. |
syuzhet | Contains functions for sentiment analysis, extracting sentiment scores from text. |
tm | Text mining package to handle text data preprocessing, including cleaning and formatting. |
Important: Ensure that you have an active internet connection while installing packages, as R needs to download them from CRAN or other repositories.
Preparing Your Text Data for Sentiment Analysis in R
Text data requires extensive preprocessing before it can be effectively used in sentiment analysis tasks. The goal is to convert raw textual data into a clean format, ensuring the removal of irrelevant elements and the extraction of meaningful information. This process allows the machine learning model to focus on the most important features of the text, improving the accuracy of sentiment classification.
In R, text preprocessing involves several critical steps. These typically include text cleaning, tokenization, stopword removal, and text normalization. Below, we discuss these steps in detail and present tools and functions commonly used in R for this purpose.
Steps for Text Data Preparation
- Text Cleaning: Remove unwanted characters, punctuation, and numbers that do not contribute to sentiment analysis. Common functions like gsub() in R can be used to clean the text.
- Tokenization: Split text into smaller units called tokens (e.g., words or phrases). The tokenizers package can be utilized to tokenize sentences or words.
- Stopword Removal: Stopwords such as "the," "is," and "in" don’t convey significant meaning and should be removed. R provides the tm package, which includes a predefined list of stopwords.
- Text Normalization: Transform text into a consistent format. This includes converting text to lowercase, stemming words (reducing words to their root form), and handling synonyms.
Useful R Libraries
- tm: A comprehensive text mining package that provides functionality for text cleaning, stopword removal, and stemming.
- textclean: Useful for cleaning and normalizing text data.
- tidytext: Integrates well with the tidyverse package and is ideal for tokenization and creating tidy text data structures.
Text preprocessing is the foundation for any successful sentiment analysis. Clean and well-processed data ensures that the model will accurately interpret the emotional tone of the text.
Example of Text Data Preparation
Original Text | Preprocessed Text |
---|---|
I love the new design! It's fantastic!! | love new design fantastic |
This is a terrible movie... | terrible movie |
Using the 'tidytext' Package for Sentiment Analysis in R
The 'tidytext' package is a powerful tool for text mining and sentiment analysis in R. By transforming text data into a tidy format, it simplifies the process of analyzing and visualizing text-based information. This package provides several functions that make it easy to calculate sentiment scores, which are essential for understanding the emotional tone of a dataset.
To analyze sentiment, the 'tidytext' package uses lexicons like "bing" and "afinn," which assign scores to words based on their positive or negative sentiment. These lexicons are directly applicable to the tidy data format, making it easier to calculate and analyze sentiment scores for large text datasets.
Key Steps to Process Sentiment Scores
- Tokenization: First, text data is tokenized, breaking it down into individual words or phrases.
- Sentiment Lexicon Application: Sentiment scores are then assigned to each word using a predefined lexicon.
- Sentiment Aggregation: Finally, sentiment scores are aggregated to provide an overall sentiment for each document or sentence.
Example Code to Calculate Sentiment Scores
library(tidytext)
library(dplyr)
# Sample text data
text_data <- tibble(line = 1:3, text = c("I love programming in R!",
"R is a bit challenging, but rewarding.",
"The syntax is confusing and frustrating."))
# Tokenize and apply sentiment lexicon
sentiment_scores <- text_data %>%
unnest_tokens(word, text) %>%
inner_join(get_sentiments("bing")) %>%
count(line, sentiment)
# View sentiment scores
sentiment_scores
Sentiment Aggregation Table
Line | Sentiment | Count |
---|---|---|
1 | positive | 1 |
2 | neutral | 1 |
3 | negative | 1 |
Sentiment analysis using the 'tidytext' package allows for efficient processing of text data, making it easy to identify emotional patterns and trends in a given dataset.
Visualizing Sentiment Distribution Using ggplot2 in R
Analyzing sentiment from text data often involves understanding the distribution of positive, negative, or neutral sentiments. In R, the ggplot2 package is an excellent tool to visualize these sentiment distributions effectively. This package allows you to create various types of plots, including bar plots, histograms, and density plots, to help identify patterns in sentiment scores across datasets.
One of the most straightforward ways to visualize sentiment analysis results is by plotting the distribution of sentiment categories. With the help of ggplot2, you can easily create a bar plot that showcases how many positive, negative, or neutral sentiments are present in your data. Below is a simple workflow for visualizing the sentiment distribution:
- Perform sentiment analysis using a sentiment lexicon or sentiment analysis model.
- Store the sentiment scores or categories in a data frame.
- Use ggplot2 to plot a bar chart that displays the frequency of each sentiment class.
Here is a sample R code for generating a sentiment distribution plot:
library(ggplot2) library(dplyr) # Sample data sentiment_data <- data.frame(sentiment = c("positive", "negative", "neutral", "positive", "negative")) # Plotting sentiment distribution ggplot(sentiment_data, aes(x = sentiment)) + geom_bar(fill = "skyblue") + labs(title = "Sentiment Distribution", x = "Sentiment", y = "Frequency")
Tip: Customize your plots with different colors and themes to make them more informative and visually appealing.
Another useful method is plotting the sentiment scores over time or across different categories. For instance, you might want to observe how sentiment changes across various days or categories. In this case, you can use a line plot or a box plot. The flexibility of ggplot2 allows you to choose the best visual representation for your sentiment analysis results.
Sentiment Category | Frequency |
---|---|
Positive | 40 |
Negative | 25 |
Neutral | 35 |
Understanding Lexicons: Selecting the Right Sentiment Dictionary
When performing sentiment analysis in R, one of the critical steps is selecting the appropriate sentiment lexicon. Lexicons are predefined lists of words associated with particular sentiment scores, which are used to gauge the sentiment of a text. The choice of lexicon can significantly affect the accuracy and relevance of the results, as different lexicons have varying approaches to categorizing sentiments and emotions.
Different lexicons come with specific strengths and weaknesses, making it essential to choose one based on the requirements of the analysis. Some lexicons are more suited for analyzing social media text, while others are designed for formal written language. Selecting the right lexicon involves understanding the type of content being analyzed and ensuring the lexicon aligns with the sentiment categories that best represent the desired output.
Key Factors in Choosing a Sentiment Lexicon
- Domain Relevance: Some lexicons are tailored for specific industries or types of text. For example, the “afinn” lexicon is suited for general sentiment analysis, while others like “Loughran-McDonald” are specialized for financial contexts.
- Granularity: Lexicons differ in their level of sentiment granularity. Some may only classify sentiment as positive or negative, while others allow for more nuanced emotional categories.
- Language Support: Not all lexicons support multiple languages, so it is important to choose one that corresponds to the language of the dataset.
Popular Sentiment Lexicons
- AFINN: A lexicon focused on assigning sentiment scores to words, with a numeric scale from -5 (negative) to +5 (positive).
- bing: A lexicon that categorizes words as either positive or negative, ideal for basic polarity analysis.
- nrc: A lexicon that assigns words to emotions like anger, joy, sadness, and surprise, offering a deeper insight into sentiment.
Choosing the wrong lexicon can lead to misleading sentiment scores, making it crucial to match the lexicon to the task at hand.
Comparison of Lexicons
Lexicon | Type | Granularity | Languages Supported |
---|---|---|---|
AFINN | Word-based, numeric | Fine-grained (scores from -5 to +5) | English |
bing | Word-based, binary | Coarse (positive/negative) | English |
nrc | Emotion-based | Emotion-specific (anger, joy, etc.) | English |
Handling Different Languages and Dialects in Sentiment Analysis
When performing sentiment analysis, one of the major challenges is dealing with the variety of languages and dialects that exist in the data. Each language has its own structure, grammar, and expressions, which can complicate the task of accurately identifying sentiment. For instance, while certain words or phrases may convey negative or positive emotions in one language, the same words may carry different connotations in another. This variation requires an adaptable approach to natural language processing (NLP) techniques to account for linguistic diversity.
Furthermore, dialects within a single language present an additional layer of complexity. For example, English spoken in the United States differs significantly from that spoken in the United Kingdom or Australia. These variations can affect word usage, slang, and cultural references that influence sentiment. As a result, sentiment analysis models need to be trained with data that reflects these differences to ensure they provide accurate results across diverse linguistic contexts.
Key Considerations for Multilingual Sentiment Analysis
- Language Detection: Before applying sentiment analysis, it's crucial to detect the language of the text. Automatic language detection models can help categorize the input, ensuring the correct linguistic model is used.
- Preprocessing: Text normalization (such as stemming and lemmatization) must be done separately for each language to account for unique morphological features.
- Custom Lexicons: Sentiment lexicons may need to be customized for each language, as words expressing sentiment may not directly translate across languages.
Challenges in Dialect Handling
- Cultural Context: Different dialects often reflect specific cultural contexts, making it important to understand local references and idioms that may influence sentiment.
- Slang and Informal Speech: Dialects often introduce slang and informal expressions that may not be captured in standard language models.
- Regional Variations: Words that are considered positive or neutral in one region may be perceived as negative or offensive in another.
Example of Language-Specific Sentiment Analysis
Language | Positive Word | Negative Word |
---|---|---|
English | Happy | Sad |
Spanish | Feliz | Triste |
French | Heureux | Triste |
Adapting sentiment analysis models to handle multiple languages and dialects requires careful attention to linguistic nuances, regional variations, and cultural context. By employing appropriate techniques, accurate sentiment detection can be achieved even in the most diverse datasets.
Optimizing Sentiment Analysis Performance on Large Datasets
Sentiment analysis is a fundamental task in Natural Language Processing (NLP), especially when dealing with large volumes of data. As datasets grow in size, ensuring that sentiment models run efficiently becomes a critical aspect of the analysis. Several techniques can be employed to optimize performance while maintaining the quality of sentiment classification.
One of the primary challenges when working with large datasets is computational efficiency. A combination of dimensionality reduction, model selection, and parallelization can significantly improve the processing time. Below are some key approaches to consider for optimizing sentiment analysis on large datasets:
Key Techniques for Optimization
- Dimensionality Reduction: Reducing the feature space can help improve the speed of training and prediction. Methods like Principal Component Analysis (PCA) or Term Frequency-Inverse Document Frequency (TF-IDF) weighting can help by eliminating redundant or irrelevant features.
- Model Simplification: Using simpler models such as Logistic Regression or Naive Bayes instead of complex ones like Deep Neural Networks can provide faster results, especially for large-scale data processing.
- Parallel Processing: Splitting the data and running models on multiple cores or distributed systems (e.g., using Hadoop or Spark) can dramatically speed up computation.
- Transfer Learning: Instead of training a model from scratch, utilizing pre-trained models (e.g., BERT or GPT) and fine-tuning them for specific sentiment tasks can save time and resources.
Steps to Optimize Performance
- Preprocessing: Clean and preprocess the data by removing stop words, stemming, and lemmatization. This ensures that the model only processes meaningful words, improving accuracy and speed.
- Feature Engineering: Use features like n-grams, word embeddings, or sentiment lexicons to enhance model understanding while reducing unnecessary complexity.
- Model Evaluation: Continuously evaluate model performance using cross-validation and other metrics like accuracy, precision, recall, and F1 score to ensure optimal balance between speed and accuracy.
Optimizing sentiment analysis for large datasets is not just about faster execution but also about maintaining a balance between computational efficiency and model accuracy.
Comparison of Approaches
Technique | Impact on Performance | Key Advantage |
---|---|---|
Dimensionality Reduction | Reduces feature space, improving speed | Faster processing with minimal loss in accuracy |
Parallel Processing | Distributes workload across multiple cores | Significantly reduces time for large datasets |
Transfer Learning | Reduces training time by leveraging pre-trained models | High accuracy with lower computational costs |
Interpreting and Acting on Sentiment Analysis Results
Sentiment analysis allows organizations to evaluate public perception of their products, services, or brands. Once sentiment data is collected, it is crucial to interpret it accurately to drive decisions and actions. A key aspect of interpretation is understanding the distribution of sentiment, whether it is positive, negative, or neutral. This breakdown helps identify the root causes of customer emotions and facilitates better-targeted responses.
After gathering sentiment analysis results, the next step is translating those insights into actions. Whether improving customer satisfaction, addressing product flaws, or enhancing marketing strategies, acting on sentiment data ensures that businesses remain agile and responsive to their audience's needs.
Steps for Interpreting Sentiment Data
- Data Preparation: Cleanse the data by removing irrelevant content, noise, or outliers that might distort the analysis.
- Sentiment Distribution: Examine the percentage of positive, negative, and neutral sentiments to understand overall sentiment trends.
- Contextual Analysis: Identify the context surrounding positive or negative sentiments to uncover the reasons behind customer emotions.
Key Actions to Take from Sentiment Insights
- Customer Support Enhancements: If negative sentiments are related to product issues, improve customer service to address concerns.
- Marketing Strategies: Leverage positive sentiment to create targeted campaigns that amplify favorable customer feedback.
- Product Development: Act on negative sentiment insights to improve or innovate products that fail to meet customer expectations.
Note: Always consider the scale of sentiment data; one isolated negative comment may not justify major operational changes, but consistent negative feedback may indicate a serious issue.
Sentiment Analysis Example
Sentiment | Percentage | Actions |
---|---|---|
Positive | 60% | Leverage in marketing campaigns and enhance customer loyalty programs. |
Negative | 25% | Address product or service issues and improve customer support channels. |
Neutral | 15% | Monitor closely to detect any emerging trends or changes in sentiment. |