Integration in Python
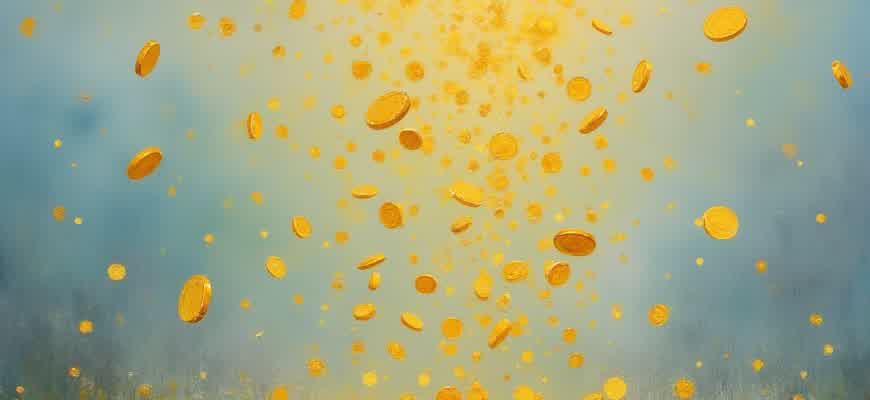
Python provides a variety of ways to integrate with external systems, APIs, and services. This flexibility is essential for creating applications that can communicate with other software and data sources. Integration can be achieved using various libraries and protocols that help bridge the gap between different environments and technologies.
Types of Integration in Python:
- API Integration: Allows Python to interact with web services using RESTful or SOAP protocols.
- Database Integration: Connects Python applications to relational and NoSQL databases using libraries like SQLAlchemy and PyMongo.
- File System Integration: Facilitates interaction with local or remote file systems for data exchange.
Popular Python Libraries for Integration:
- Requests – For making HTTP requests to external APIs.
- Pandas – Used for data manipulation, often in conjunction with external data sources.
- Celery – A distributed task queue system that integrates with Python for handling asynchronous operations.
"Integration in Python is not just about connecting systems; it's about ensuring smooth, efficient data flow and seamless communication between applications."
Common Protocols and Methods:
Protocol | Description |
---|---|
HTTP | Widely used for web-based integration and API communication. |
FTP | Used for file transfers between systems. |
WebSockets | Provides real-time, two-way communication between Python applications and other services. |
Automating Data Transfer Between APIs Using Python
Automating data transfer between APIs is a common practice to enhance the workflow of software systems. By integrating different services, Python developers can save time and avoid errors that occur during manual data handling. The process typically involves extracting data from one API, transforming it as necessary, and sending it to another API. Python's vast ecosystem of libraries simplifies this process, making it a go-to solution for API interaction.
Python offers several tools for automating data exchange, such as the requests library for handling HTTP requests, and JSON for working with data formats. Integration can be performed in various ways depending on the complexity and requirements of the task, including direct communication, scheduled transfers, or event-driven triggers. Below are some best practices and common approaches to achieve this automation:
Key Steps for API Integration
- Request Data: Use the requests library to fetch data from the source API.
- Transform Data: If necessary, modify the data to match the required format of the target API.
- Send Data: Use the same requests library to post the transformed data to the destination API.
- Handle Errors: Ensure proper error handling, such as retries or logging, in case of failure during the exchange.
Example Workflow
Step | Action |
---|---|
1 | Send a GET request to the source API to retrieve data. |
2 | Parse the response and transform the data into the required format. |
3 | Send a POST request to the destination API with the formatted data. |
Note: When automating data exchange, always ensure that rate limits and API authentication mechanisms are handled properly to avoid service disruptions.
Best Practices
- Secure API Keys: Store API keys securely using environment variables or a secrets manager.
- Error Logging: Implement robust logging to track issues and monitor data exchange.
- Rate Limiting: Respect API rate limits to prevent overloading services.
Setting Up Database Connections in Python for Real-Time Applications
Establishing an efficient and reliable database connection is crucial for real-time systems that handle constant data influx, such as financial applications or live chat services. In Python, various libraries like `psycopg2` for PostgreSQL, `PyMySQL` for MySQL, and `SQLAlchemy` for ORM-based interactions can be used to ensure that real-time updates are processed without delays or failures.
In this context, selecting the right connection strategy and understanding the core aspects of persistent connections is vital. Real-time applications often require optimized database access to minimize latency and maximize throughput. This is especially true when dealing with massive amounts of concurrent data requests, where even a small delay can significantly affect user experience.
Setting Up Persistent Database Connections
To ensure continuous data flow in a real-time application, using persistent connections is highly recommended. Persistent connections are maintained throughout the lifecycle of the application, reducing the need to repeatedly open and close connections, which can be costly in terms of performance. Below are the key components to keep in mind while setting up persistent database connections in Python:
- Connection Pooling: Use libraries like `SQLAlchemy` or `psycopg2` with pooling to maintain a pool of open connections, making it easier to reuse connections instead of establishing a new one each time.
- Database Cursor Management: Use cursors effectively to handle large data sets in a memory-efficient manner. Closing cursors properly after use ensures that resources are released.
- Timeouts and Reconnect Strategies: Implement reconnect logic and configure appropriate timeouts for database connections to avoid application downtime during brief network or database failures.
Best Practices for Real-Time Database Connections
In a high-performance environment, managing database connections efficiently is not optional but essential. It's recommended to limit the number of connections per user and monitor database health periodically to prevent bottlenecks.
- Set appropriate database connection limits based on the load your application expects.
- Implement exponential backoff strategies to handle retrying failed connections.
- Use read replicas or caching layers (e.g., Redis) for offloading read-heavy operations.
Sample Database Connection Setup
Here's an example of how to set up a PostgreSQL connection using `psycopg2` with connection pooling:
Code Snippet |
---|
import psycopg2 from psycopg2 import pool # Set up a connection pool connection_pool = psycopg2.pool.SimpleConnectionPool( 1, 20, user="your_user", password="your_password", host="localhost", port="5432", database="your_db" ) # Get a connection from the pool conn = connection_pool.getconn() # Use the connection cursor = conn.cursor() cursor.execute("SELECT * FROM your_table;") data = cursor.fetchall() # Return the connection to the pool connection_pool.putconn(conn) |
Handling File Transfers and Synchronization with Python Scripts
Managing file transfers and synchronizing data between systems is a critical task in various automation scenarios. Python provides robust libraries that simplify these processes, ensuring reliable and efficient file handling. By leveraging tools like FTP, SFTP, and cloud storage APIs, developers can seamlessly integrate file transfers into their applications.
Synchronizing files across different systems or directories involves tracking changes and ensuring consistency. Python scripts can be used to automate this process by comparing file timestamps, checksums, or metadata. Tools like `rsync` or `shutil` can aid in creating backup scripts or setting up sync operations between servers and local machines.
Key Concepts in File Transfer and Synchronization
- FTP (File Transfer Protocol): A common method for transferring files over a network.
- SFTP (Secure File Transfer Protocol): A secure alternative to FTP, ensuring encrypted communication.
- Cloud Storage APIs: Interfaces for managing files stored in cloud services like Google Drive or AWS S3.
- File Hashing: A technique for comparing files by generating unique checksums to detect differences.
Steps for Automating File Synchronization
- Set Up FTP/SFTP Connection: Use libraries like `ftplib` or `paramiko` to establish connections for secure file transfer.
- Compare File States: Check timestamps, file size, or hashes to determine which files need to be updated.
- Transfer and Sync: Use `shutil` for local copying or `paramiko` for remote file handling to sync the files.
- Handle Errors and Logging: Implement error handling and log actions for troubleshooting and tracking progress.
Example of Python Script for SFTP File Transfer
import paramiko def sftp_transfer(local_path, remote_path, host, username, password): try: # Establish an SFTP connection transport = paramiko.Transport((host, 22)) transport.connect(username=username, password=password) sftp = paramiko.SFTPClient.from_transport(transport) # Transfer the file sftp.put(local_path, remote_path) sftp.close() transport.close() print("File transferred successfully!") except Exception as e: print(f"Error during transfer: {e}") # Usage sftp_transfer('local_file.txt', '/remote/dir/file.txt', 'example.com', 'user', 'password')
Note: Always use secure methods like SFTP when transferring sensitive data to avoid potential security risks.
Comparison of File Transfer Methods
Protocol | Security | Use Case |
---|---|---|
FTP | Low (unencrypted) | Simple, non-sensitive transfers |
SFTP | High (encrypted) | Secure file transfers over the internet |
Cloud Storage API | Depends on provider | Cloud backups, remote access |
Integrating External Services in Python Web Applications
When building Python-based web applications, integrating third-party services is often necessary to enhance the functionality of the application. These services could range from payment gateways, authentication providers, to email services or cloud storage. Utilizing external APIs allows developers to avoid reinventing the wheel and to focus on core application features.
Integration with third-party services can be accomplished through RESTful APIs, SDKs, or direct communication with the service provider's libraries. This process involves careful management of API keys, security concerns, and error handling to ensure seamless operation within your web application.
Steps to Integrate Third-Party Services
- Identify the service and review its documentation.
- Obtain necessary credentials (e.g., API keys or OAuth tokens).
- Set up a connection to the service's API using a Python library (e.g., Requests or HTTP client).
- Implement appropriate error handling for API failures or timeouts.
- Test the integration thoroughly to ensure data is passed correctly.
Example: Payment Gateway Integration
A typical integration with a payment gateway like Stripe involves creating a customer, processing payments, and handling webhooks for payment confirmation. The service documentation will provide Python SDKs or examples of making HTTP requests to their endpoints.
Key Considerations
- Authentication & Security: Always use environment variables to store sensitive information like API keys and tokens.
- Error Handling: Ensure your application can gracefully handle issues such as rate limits or downtime from third-party services.
- Data Privacy: Ensure compliance with regulations like GDPR when sending and receiving user data to/from external services.
Example Integration: Email Service
Many developers use services like SendGrid for email delivery in their Python applications. Below is a brief example of how such an integration might look:
Step | Action | |
---|---|---|
1 | Install the SendGrid Python library: pip install sendgrid |
|
2 | Set up your SendGrid API key in environment variables. | |
3 | Use the API to send an email: | from sendgrid import SendGridAPIClient from sendgrid.helpers.mail import Mail message = Mail(from_email='[email protected]', to_emails='[email protected]', subject='Test', html_content='Test Email') sg = SendGridAPIClient('YOUR_API_KEY') response = sg.send(message) |
Building Custom Python Modules for Seamless Software Integration
Creating custom Python modules is a crucial step in ensuring smooth and efficient software integration, particularly when dealing with multiple systems or services. By developing tailored modules, developers can extend Python’s capabilities to suit specific project requirements, thus enhancing compatibility with various data sources, APIs, or platforms. This approach eliminates the need for excessive dependencies on third-party libraries, enabling better control over functionality and performance.
When crafting a Python module, it is essential to keep in mind how it will interact with other components of the software ecosystem. A well-designed custom module should be easily integrable, modular, and adaptable, which allows the software to scale or evolve without introducing complexity or redundancy. Below are some key considerations when building such modules.
Key Considerations
- Modular Design: Break down complex functionality into smaller, reusable components that can be combined to build larger features.
- API Compatibility: Ensure that your module can communicate smoothly with external APIs or services, using appropriate authentication and data formats.
- Error Handling: Implement robust error handling to deal with issues like network failures, invalid data, or unexpected conditions.
- Testing and Debugging: Thoroughly test the module in isolation and within the context of the larger application to ensure proper functionality.
“A well-structured Python module can be the cornerstone of an efficient and maintainable software system, ensuring that integration tasks are simplified and future updates are easier to manage.”
Structure of a Custom Python Module
- Define Functionality: Clearly outline the purpose and the core functionality of the module. For example, whether it's for data manipulation, API communication, or other tasks.
- Write Modular Code: Split the code into smaller functions or classes to ensure reusability and clarity.
- Testing: Use Python’s built-in unit testing framework or other tools to verify the module’s behavior in various scenarios.
- Documentation: Provide detailed documentation and usage examples to facilitate integration by other developers or teams.
Example Module Structure
File | Description |
---|---|
module.py | Main module file containing core functionality. |
tests.py | Test file to ensure the module works as expected. |
README.md | Documentation file with usage instructions and examples. |
Optimizing Python Code for External System Integration
When integrating Python with external systems such as databases, APIs, or hardware interfaces, it's essential to enhance the performance and reliability of the communication. Efficient code execution minimizes latency, reduces overhead, and ensures smooth data exchanges. There are several strategies to optimize Python's performance when working with external systems, ranging from proper error handling to utilizing asynchronous operations.
Python provides several tools and libraries to facilitate such integrations. By using asynchronous programming techniques, optimizing data transfer protocols, and leveraging specialized libraries, Python developers can achieve fast and scalable interactions with external systems. Below are key techniques to consider.
Key Strategies for Optimization
- Asynchronous Programming: Use async/await to handle I/O-bound tasks without blocking execution. This is especially useful when interfacing with external APIs or databases where waiting for a response can be a bottleneck.
- Efficient Data Serialization: Choose the most efficient data serialization formats like Protocol Buffers or MessagePack over JSON for faster parsing and reduced network overhead.
- Connection Pooling: Reuse database or API connections to avoid the overhead of repeatedly opening and closing connections, significantly improving performance in high-frequency requests.
- Batching Requests: Group multiple requests into a single operation, reducing the number of round trips to external systems and minimizing latency.
Optimizing External System Interactions
- Use Efficient Libraries: Libraries such as asyncio and aiohttp can dramatically reduce the overhead of handling multiple simultaneous connections.
- Limit Data Transfer: Only request or send the data that is necessary. Reducing payload size improves transfer speeds and reduces resource consumption.
- Timeouts and Retries: Implement appropriate timeouts for requests and set retry strategies to handle transient failures effectively, avoiding unnecessary waiting times and resource blocking.
Performance Comparison Table
Method | Performance Benefit | Use Case |
---|---|---|
Asynchronous I/O | Improved concurrency and reduced wait time | API calls, database queries |
Connection Pooling | Reduced connection overhead, faster repeated access | Database, Web API |
Batch Processing | Reduced round trips, lower network overhead | Multiple small requests |
Tip: Always ensure that external system integration is designed for scalability. Consider implementing rate-limiting or throttling mechanisms to handle increased loads without overloading the external system.
Testing and Debugging Integration Processes in Python
Ensuring that your integration workflows in Python function correctly is essential for seamless system interactions. Integration testing helps verify that the combined modules perform as expected when integrated into a complete system. However, debugging these processes can be challenging due to the complexity of dependencies, communication between systems, and variable input conditions. Effective testing and debugging strategies are crucial to maintain the reliability of integrated solutions.
Python offers various tools and methods to streamline testing and debugging workflows. By using unit tests, logging, and step-by-step debugging techniques, developers can efficiently identify issues within their integration workflows. Below are some useful strategies to consider for testing and debugging integration processes in Python.
Key Strategies for Testing Integration Workflows
- Unit Testing: Break down the integration points into smaller, testable components. This allows you to isolate and test individual units before combining them in the larger workflow.
- Mocking External Services: When integrating with external APIs or services, use mocking libraries like unittest.mock to simulate responses and ensure your integration behaves as expected in various scenarios.
- End-to-End Testing: Once individual components are validated, perform end-to-end tests to verify the entire integration pipeline. Use testing frameworks like pytest or nose for comprehensive testing.
Debugging Integration Issues
Debugging integration workflows in Python often requires systematic approaches to isolate issues in large systems. When dealing with multiple components, the debugging process becomes intricate, and tracking down the source of an error requires patience and the use of suitable tools.
- Logging: Implement logging using Python's built-in logging module to capture detailed execution traces and error messages. This helps identify where the issue arises in the workflow.
- Interactive Debugging: Use the pdb debugger for interactive, step-by-step inspection of code execution. This allows you to set breakpoints and inspect variables to understand the flow and pinpoint failures.
- Exception Handling: Properly handle exceptions throughout your integration code. By using try-except blocks effectively, you can provide meaningful error messages that assist in debugging.
Common Challenges in Integration Testing and Debugging
Challenge | Solution |
---|---|
Complex Dependencies | Use mocking to simulate external dependencies and focus on testing the integration logic. |
Non-Deterministic Inputs | Control inputs by using fixtures or mock data to ensure consistent test results. |
Hidden Errors in External Services | Log responses and timeouts from external services to identify intermittent issues. |
Note: Always ensure that your integration tests run in an environment similar to production to catch issues early in the development cycle.