Integration in Javascript
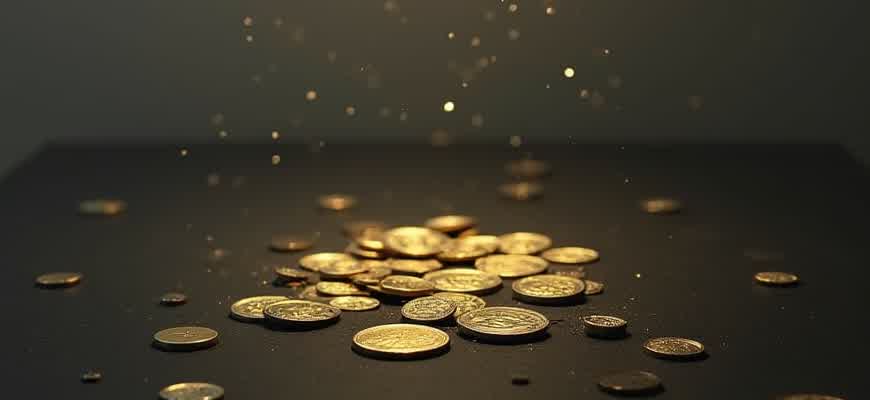
Setting Up API Integration with JavaScript
Integrating an API into a JavaScript application involves making HTTP requests to retrieve or send data between your application and a remote server. The process typically includes setting up the request, handling responses, and managing potential errors or edge cases. Below is an overview of how to effectively connect an API to your JavaScript code.
To begin with, understanding how to work with different methods of HTTP requests is essential. In most cases, you will interact with APIs using GET, POST, PUT, or DELETE methods. Using the Fetch API is a modern approach for making these requests, providing a flexible and clean solution for handling HTTP communication in JavaScript.
Steps to Set Up API Integration
- Use the Fetch API to initiate a request to an endpoint.
- Handle the response asynchronously using promises or async/await.
- Parse the response data (usually in JSON format) and integrate it into your app.
- Handle errors and edge cases, such as failed network requests or invalid data.
Here is an example of a basic API request setup:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error:', error));
Note: The Fetch API returns a promise, so always use `.then()` or async/await for handling the response and errors.
Error Handling
Error handling is crucial when working with API integrations. A common approach is to check for a successful HTTP response status (like 200 OK) and handle any other status codes accordingly. For instance, if the request fails, the application should display a user-friendly message or attempt a retry.
- Check for a successful response (status 200).
- If the status is not OK, display an error message or take corrective actions.
- Use try-catch blocks for handling asynchronous errors, especially when using async/await.
Sample Code with Error Handling
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); if (!response.ok) { throw new Error('Network response was not ok'); } const data = await response.json(); console.log(data); } catch (error) { console.error('There was a problem with the fetch operation:', error); } }
Important: Always validate the data before integrating it into your application to prevent issues like invalid or incomplete data affecting the functionality.
API Response Format
Many APIs return data in JSON format, which can be easily parsed in JavaScript. It’s important to understand the structure of the response data so you can extract and use it effectively in your application.
Field | Type | Description |
---|---|---|
id | Integer | Unique identifier for the item |
name | String | Name of the item |
price | Float | Price of the item |
How to Use Fetch for Smooth Data Handling in JavaScript
JavaScript's Fetch API provides a powerful way to interact with data over the network, making it a key tool for web developers. With its promise-based structure, Fetch allows for cleaner and more readable asynchronous code when dealing with external APIs or server resources. Unlike older methods like XMLHttpRequest, Fetch provides an easy-to-use syntax and a more flexible approach for handling both requests and responses.
Fetching data with Fetch involves several steps: making the request, handling the response, and managing any errors that occur. Below, we will explore how to implement each of these steps and ensure smooth integration with your JavaScript applications.
Making a Simple Fetch Request
To start, use the fetch() method, which returns a promise. You can pass the URL of the resource you want to fetch as an argument:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error('Error fetching data:', error));
In the above example, response.json() converts the response body into a JSON object. Handling errors using catch() ensures that if anything goes wrong, an error message is logged to the console.
Handling Multiple Requests
If your application requires fetching multiple resources at once, you can use the Promise.all() method to handle multiple fetch requests in parallel:
Promise.all([ fetch('https://api.example.com/data1'), fetch('https://api.example.com/data2') ]) .then(responses => Promise.all(responses.map(response => response.json()))) .then(data => { console.log(data[0]); // Data from the first API console.log(data[1]); // Data from the second API }) .catch(error => console.error('Error fetching data:', error));
Best Practices for Fetching Data
When using Fetch, it's essential to follow certain practices to ensure efficient data handling:
- Always handle errors: Use catch() to capture network or parsing errors.
- Consider response validation: Check if the response status is successful (status code 200) before parsing the data.
- Use async/await: For cleaner, more readable code, consider using async/await for handling asynchronous requests.
Response Status Codes
While working with Fetch, it's important to handle different HTTP status codes appropriately. Here’s a simple table that illustrates how to handle various status codes:
Status Code | Meaning | Action |
---|---|---|
200 | OK | Proceed with the response data. |
404 | Not Found | Handle the error and display a message. |
500 | Server Error | Show an error message or retry. |
Always validate response status before proceeding with parsing the response body. Failing to do so may result in unexpected behavior or runtime errors.
Error Handling Strategies in JavaScript API Integration
When integrating with APIs in JavaScript, proper error handling is essential for maintaining application reliability and improving user experience. API calls can fail for various reasons, such as network issues, timeouts, or invalid data responses. Therefore, adopting a comprehensive approach to handle errors is crucial to ensure that your application behaves predictably under all circumstances.
To manage errors effectively, developers often rely on different strategies, including try-catch blocks, error status codes, and fallback mechanisms. Implementing robust error-handling patterns can greatly enhance the stability and resilience of the application while ensuring that issues are reported or handled in a user-friendly manner.
Key Error Handling Approaches
- Try-Catch Blocks: These are used to catch synchronous and asynchronous errors that may arise during API calls. Wrapping the API call in a try block ensures that any error is caught and can be processed or logged.
- Promise .catch() Method: When working with promises, the .catch() method allows you to handle rejected promises, offering a way to manage errors in asynchronous code.
- Status Code Verification: After receiving a response from an API, checking the HTTP status code is vital to determine if the request was successful or if an error occurred (e.g., 404, 500).
Handling Different Types of Errors
- Network Errors: These occur when the request fails due to network connectivity issues. They can be handled by retrying the request or displaying an error message to the user.
- Timeout Errors: Timeouts happen when an API takes too long to respond. Implementing timeouts in your request configuration can allow for retries or alternate actions.
- API Response Errors: Even if a request succeeds, the response might contain errors. Always check for specific error codes or messages in the response body to handle API-related issues properly.
Important: Always provide users with clear and concise error messages to prevent confusion and improve the user experience when something goes wrong.
Example of Error Handling with Fetch API
Code Example | Description |
---|---|
fetch('https://api.example.com/data') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .then(data => console.log(data)) .catch(error => console.error('There was a problem with the fetch operation:', error)); |
Using the fetch API, the code checks if the response is successful and throws an error if not. Errors are caught and logged with the .catch() method. |
Optimizing Performance in JavaScript Data Integrations
When integrating data in JavaScript applications, performance is a key concern, especially when dealing with large datasets or real-time data processing. Efficient data handling can significantly impact the responsiveness and overall performance of the application. It is crucial to implement strategies that minimize processing time and resource consumption while ensuring data accuracy.
Several optimization techniques can help improve performance in JavaScript integrations. These methods focus on reducing the complexity of operations, optimizing memory usage, and minimizing the number of reflows and repaints in the DOM. Let's explore some of the best practices for optimizing data integrations in JavaScript.
Techniques for Enhancing Performance
- Asynchronous Data Fetching: Use asynchronous methods such as Promises or async/await to handle data fetching. This ensures that the application remains responsive while the data is being loaded in the background.
- Debouncing and Throttling: Limit the frequency of data requests or processing by using debouncing and throttling techniques. This prevents unnecessary calls to APIs or redundant computations, improving efficiency.
- Efficient Data Storage: Store data in a format that minimizes memory usage, such as using Map or Set for large datasets, which have better lookup times compared to arrays or objects.
Best Practices for Reducing Computational Overhead
- Minimize DOM manipulations. Every time the DOM is updated, it triggers reflows and repaints, which can be costly in terms of performance. Batch DOM updates together to reduce the number of reflows.
- Use web workers to offload heavy data processing tasks to background threads, keeping the main thread free for UI updates and user interactions.
- Use virtualization when rendering large lists or tables, rendering only the visible portion of the content at any given time to reduce memory and rendering time.
Memory Management Strategies
Effective memory management is crucial when integrating large datasets. Failure to properly manage memory can result in performance degradation and potential memory leaks.
Strategy | Description |
---|---|
Garbage Collection | Ensure proper garbage collection by eliminating references to unused objects, allowing the garbage collector to reclaim memory. |
Object Pooling | Reuse objects from a pre-allocated pool instead of frequently creating and destroying them, reducing overhead. |
Memory Profiling | Use memory profiling tools to identify memory bottlenecks and optimize the allocation and deallocation of resources. |
Effective memory management and minimizing the computational complexity of data integrations are essential for achieving optimal performance in JavaScript applications.
Integrating Webhooks for Real-Time Data in JavaScript
Webhooks allow for real-time communication between servers and client applications. They enable instant data transfer by sending HTTP POST requests to a specified endpoint whenever a predefined event occurs. This approach is highly useful for applications that need to respond to changes or updates in external systems, such as payment gateways, messaging services, or IoT devices.
JavaScript provides several ways to handle webhooks effectively. By utilizing server-side frameworks or libraries like Express.js, developers can easily create endpoints to capture and process webhook notifications. Once the webhook data is received, it can be processed and stored in a database or used to trigger further actions within the application.
Steps to Integrate Webhooks in JavaScript
- Set up a webhook endpoint: Create a route on your server that listens for incoming POST requests from the source service.
- Validate the request: Check if the incoming request is from a trusted source by validating the signature or token included in the webhook.
- Process the data: Parse the payload from the webhook and take appropriate actions, such as updating a user interface or triggering other APIs.
- Respond to the service: Send a response back to the source service to acknowledge the receipt of the webhook and indicate successful processing.
Example of a webhook payload:
{ "event": "payment_success", "data": { "amount": 100, "currency": "USD", "transaction_id": "abc123" } }
After receiving the webhook payload, you can process the data to update your application in real-time. For instance, a payment service may notify you when a transaction is completed, and you can use this data to update the user's account or send a confirmation email.
Real-time integration of webhooks enables instant responses to changes in external systems, making it ideal for applications that require immediate updates.
Webhook Integration Example in JavaScript (Node.js)
Code |
---|
const express = require('express'); const app = express(); app.use(express.json()); app.post('/webhook', (req, res) => { const data = req.body; console.log('Received webhook data:', data); res.status(200).send('Webhook received'); }); app.listen(3000, () => { console.log('Server running on port 3000'); }); |
Testing API Integrations in JavaScript with Mock Servers
When building applications that rely on external APIs, it's essential to test how the system integrates with them. Mock servers allow developers to simulate the behavior of real APIs without making actual network requests, providing a controlled environment for testing various scenarios. This approach is particularly useful when the actual API is not yet available, or when you want to isolate external dependencies during testing.
Mock servers in JavaScript can be set up using various tools and libraries such as MSW (Mock Service Worker) or json-server. These tools enable developers to intercept requests and return predefined responses, allowing for testing under specific conditions. By using mock data, developers can ensure their application handles responses correctly, even in edge cases.
Advantages of Using Mock Servers for API Testing
- Isolation: Test cases can be executed without relying on external services, ensuring consistent results.
- Speed: Mock servers are faster than actual API calls, reducing the overall testing time.
- Flexibility: You can simulate various API behaviors, such as slow responses or errors, to test how your application handles them.
Setting Up a Mock Server
- Install a Mock Server Library: Choose a library like MSW or json-server and add it to your project.
- Configure the Routes: Define the API endpoints you want to mock, along with the responses they should return.
- Intercept API Calls: Set up the mock server to intercept the requests made by your application and return the mock responses.
- Run the Tests: Execute the tests to ensure your application handles the mocked responses correctly.
Mock servers allow for testing the full flow of your application without relying on an actual backend service, providing faster and more reliable tests.
Example: Mocking an API Response
Request | Response |
---|---|
GET /api/users | { "users": ["John", "Jane", "Doe"] } |
POST /api/users | { "status": "success" } |