Segmentation Analysis in R
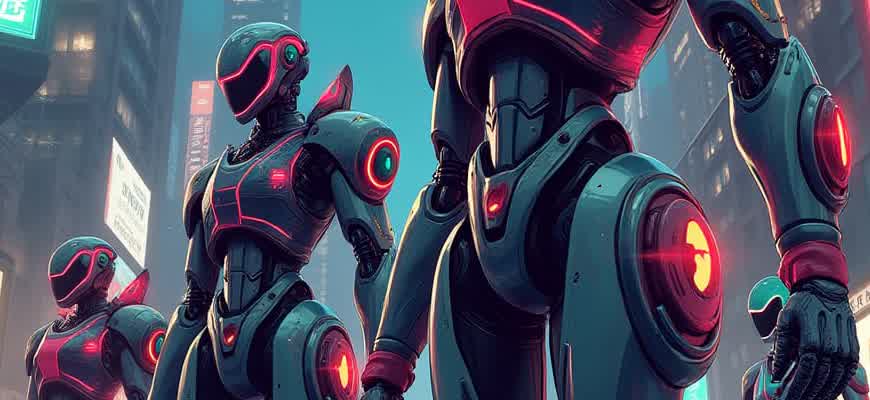
Segmentation analysis is a powerful tool in data science used to divide a dataset into distinct groups or segments based on certain characteristics. In R, this process can be accomplished using various techniques like clustering, classification, and decision trees. By identifying patterns and relationships within the data, it helps businesses and researchers target specific groups effectively for tailored strategies.
To perform segmentation analysis in R, several key steps are involved:
- Data preparation and cleaning
- Feature selection and transformation
- Choosing appropriate segmentation models
- Evaluating and interpreting the results
Important: The choice of segmentation method depends on the nature of the data and the objective of the analysis.
Here is an example of how segmentation analysis can be approached using the k-means clustering algorithm:
Step | Action |
---|---|
1 | Load and preprocess the data |
2 | Standardize features |
3 | Apply k-means clustering |
4 | Evaluate clustering results |
Data Preparation for Segmentation in R
Before performing segmentation analysis in R, it is essential to properly prepare the dataset to ensure accurate and meaningful results. The quality and structure of the data play a significant role in the effectiveness of segmentation models. A well-structured dataset allows for better insights and more reliable outcomes. The first step involves ensuring the data is clean, complete, and in the correct format for analysis. In this process, missing values, outliers, and inconsistencies must be addressed to prevent bias in segmentation outcomes.
The second step in data preparation is selecting the appropriate features for segmentation. It is crucial to choose variables that are relevant to the segmentation objective. These features can include demographic information, transactional data, or behavior patterns. Once the necessary variables are identified, they need to be preprocessed, including normalization or standardization, depending on the chosen segmentation method.
Key Steps in Data Preparation
- Data Cleaning: Identify and handle missing values, duplicates, or erroneous data entries.
- Feature Selection: Choose the most relevant features that contribute to the segmentation objective.
- Normalization or Standardization: Scale the data to ensure consistent measurement units across variables.
- Outlier Detection: Identify and treat outliers that may distort segmentation results.
Example of Data Preprocessing Steps
Data preprocessing often involves the following tasks:
- Remove duplicate records from the dataset.
- Replace or impute missing values using statistical methods or algorithms.
- Normalize or standardize continuous variables using methods like min-max scaling or z-score transformation.
- Handle categorical data by converting it into numerical format using encoding techniques like one-hot encoding.
Data Preparation Summary
Step | Action | Tools/Methods |
---|---|---|
Data Cleaning | Remove duplicates, handle missing values | R packages like dplyr, tidyr |
Feature Selection | Select relevant variables | Statistical tests, correlation analysis |
Normalization | Scale variables | scale(), min-max scaling |
Outlier Detection | Identify and handle outliers | IQR method, boxplots |
Choosing the Right Clustering Algorithm for Your Dataset
When performing segmentation analysis, selecting the appropriate clustering algorithm plays a crucial role in achieving meaningful and actionable insights. The decision largely depends on the nature of your data, its distribution, and the specific goals of your analysis. Each clustering method has distinct characteristics that make it suitable for particular types of datasets. Understanding these differences can help in making an informed choice that maximizes the relevance and accuracy of your segmentation results.
In this context, it’s important to consider factors such as data scale, density, the presence of outliers, and the interpretability of the clustering output. Below are key considerations when selecting a clustering technique for your project:
Factors to Consider When Choosing a Clustering Algorithm
- Data Shape and Size: For small, well-separated clusters, algorithms like K-means may perform well. However, for large, complex datasets, density-based approaches like DBSCAN can handle irregular shapes.
- Scalability: K-means is typically faster and more scalable for large datasets. Hierarchical methods, while informative, may struggle with scalability.
- Handling Outliers: Algorithms like DBSCAN are more robust to noise and outliers, making them a good choice when such data points exist in the dataset.
Popular Clustering Algorithms
- K-means: Ideal for spherical, well-separated clusters, efficient for large datasets. However, it requires pre-specifying the number of clusters.
- Hierarchical Clustering: Provides a dendrogram to visualize relationships. Suitable for smaller datasets but computationally expensive for larger ones.
- DBSCAN: Density-based algorithm that can find arbitrarily shaped clusters and handles noise effectively. Best for datasets with varying densities.
- Gaussian Mixture Models (GMM): A probabilistic model suitable for data with overlapping clusters. It’s more flexible than K-means and can model elliptical cluster shapes.
Choosing the right algorithm involves balancing between computational efficiency and the quality of the clusters. Experimenting with different algorithms and evaluating their results through methods like silhouette scores can provide insights into the best approach for your dataset.
Summary Table of Common Clustering Methods
Algorithm | Strengths | Limitations |
---|---|---|
K-means | Fast, scalable, works well with spherical clusters | Requires predefined number of clusters, sensitive to outliers |
Hierarchical | Visualizes cluster hierarchy, no need to predefine clusters | Computationally expensive for large datasets |
DBSCAN | Can find irregularly shaped clusters, robust to outliers | Sensitive to parameters like epsilon and minPts |
GMM | Flexible, handles overlapping clusters | Computationally intensive, assumes Gaussian distributions |
Implementing K-Means Clustering in R: A Detailed Guide
Clustering is a common technique used in segmentation analysis to identify patterns within a dataset. One popular method for clustering is K-means, which partitions data into a predefined number of groups based on similarity. In R, implementing K-means clustering can be done efficiently with the built-in function kmeans(). This approach allows you to categorize data into clusters based on their features, making it an essential tool for understanding the underlying structure of your data.
To begin, K-means clustering requires defining the number of clusters, which is often determined using domain knowledge or optimization methods like the Elbow Method. Once the number of clusters is specified, the algorithm assigns each data point to the nearest cluster center (centroid) and iteratively adjusts the centroids to minimize within-cluster variance.
Steps for Implementing K-Means in R
Follow these steps to perform K-means clustering in R:
- Prepare Your Data: Clean and normalize the data if necessary. K-means is sensitive to scale, so ensure that all features are on similar scales.
- Choose the Number of Clusters: Use methods like the Elbow Method to determine the optimal number of clusters.
- Apply the K-Means Algorithm: Use the
kmeans()
function in R to perform clustering. - Evaluate the Results: Analyze the clustering output, including centroids, cluster assignments, and within-cluster sum of squares.
Tip: It's essential to standardize your data before running K-means, especially when your dataset contains features with varying units or ranges.
Example Code
The following R code demonstrates how to perform K-means clustering on a sample dataset:
# Load necessary library
library(datasets)
# Example data: iris dataset
data(iris)
# Scale the data (excluding the species column)
scaled_data <- scale(iris[, -5])
# Run K-means clustering with 3 clusters
set.seed(123) # For reproducibility
kmeans_result <- kmeans(scaled_data, centers = 3)
# Output the results
print(kmeans_result)
The output of the kmeans()
function will provide cluster assignments, centroids, and the total within-cluster sum of squares.
Understanding the Output
Once the clustering is complete, the results include several important components:
Output Component | Description |
---|---|
Cluster Centers | The coordinates of the centroids of the clusters. |
Cluster Assignments | A vector of integers indicating which cluster each data point belongs to. |
Within-cluster Sum of Squares | A measure of how compact the clusters are, with lower values indicating better clustering. |
Note: It's recommended to run the algorithm multiple times with different initial centroids to avoid local minima, which can lead to suboptimal clustering results.
Understanding Hierarchical Clustering and Its Use Cases
Hierarchical clustering is a method of cluster analysis that builds a hierarchy of clusters based on the similarity between the data points. This approach does not require a predefined number of clusters, making it particularly useful in exploratory data analysis. The hierarchical structure is created by either successively merging small clusters into larger ones (agglomerative approach) or by progressively splitting a large cluster into smaller ones (divisive approach).
One of the main advantages of hierarchical clustering is its ability to provide a dendrogram–a tree-like diagram that visualizes the merging or splitting process. This allows users to determine the optimal number of clusters by cutting the tree at a desired level. It is highly effective in scenarios where understanding the relationships and structure of the data is crucial.
Key Features of Hierarchical Clustering
- Flexible number of clusters: Unlike k-means clustering, hierarchical clustering does not require specifying the number of clusters in advance.
- Dendrogram: The dendrogram provides a clear visual representation of how data points are grouped at each stage.
- Versatile distance metrics: It allows for the use of various distance measures (e.g., Euclidean, Manhattan, cosine) depending on the problem's nature.
Use Cases
- Market Segmentation: Hierarchical clustering can be applied to group customers with similar purchasing behavior, enabling targeted marketing strategies.
- Genomic Research: It is used to cluster genes with similar expression profiles, aiding in the identification of gene groups with related functions.
- Document Classification: In natural language processing, hierarchical clustering can help group similar documents based on their content.
Comparison with Other Clustering Methods
Feature | Hierarchical Clustering | K-means Clustering |
---|---|---|
Number of Clusters | No need to predefine | Must define in advance |
Cluster Structure | Tree-like (dendrogram) | Flat clusters |
Distance Metric | Flexible (Euclidean, Manhattan, etc.) | Usually Euclidean |
Hierarchical clustering is particularly useful when the relationships between data points need to be explored and understood in a nested or layered fashion.
Evaluating Cluster Quality Using Silhouette Score and Other Metrics
Assessing the quality of clustering results is crucial for understanding how well the data has been grouped. Various methods can be used to measure the coherence of clusters, among which the Silhouette Score stands out due to its ability to evaluate both cohesion and separation. The Silhouette Score ranges from -1 to 1, where a higher score indicates well-formed clusters. Additionally, there are other metrics, such as the Davies-Bouldin Index and Dunn's Index, that offer further insights into the quality of clustering solutions.
In practice, cluster evaluation metrics provide a quantitative means to compare different clustering models and choose the optimal one. Each metric has its own strengths, and they should be used in combination to get a comprehensive understanding of the clustering quality. Below are some of the most widely used metrics and how they contribute to evaluating the clustering results.
Key Metrics for Cluster Evaluation
- Silhouette Score: Measures the similarity of an object to its own cluster compared to other clusters. A value closer to 1 indicates well-separated clusters.
- Davies-Bouldin Index: Measures the average similarity ratio of each cluster with the cluster that is most similar to it. A lower score indicates better clustering.
- Dunn's Index: Focuses on the ratio of the minimum distance between clusters to the maximum intra-cluster distance. A higher value suggests better clustering.
Comparing Clustering Results
- Step 1: Calculate the Silhouette Score for all clusters.
- Step 2: Compute additional metrics such as the Davies-Bouldin Index or Dunn's Index.
- Step 3: Compare the scores across different models to determine which one provides the best clustering.
- Step 4: Validate the results using external validation metrics, such as purity or NMI, to check consistency with ground truth (if available).
Note: No single metric is definitive. It is essential to analyze multiple metrics in conjunction to form a well-rounded evaluation of clustering quality.
Example: Comparing Silhouette and Davies-Bouldin Index
Metric | Cluster A | Cluster B | Cluster C |
---|---|---|---|
Silhouette Score | 0.76 | 0.42 | 0.58 |
Davies-Bouldin Index | 0.35 | 0.68 | 0.45 |
Visualizing Clusters in R: Tools and Techniques
Effective cluster visualization is essential for understanding the structure and relationships within the data. In R, there are several methods for displaying clusters, allowing analysts to explore data patterns and validate clustering outcomes. Visual representations can help to identify distinct groups, understand their characteristics, and assess the performance of clustering algorithms.
Various R packages provide powerful tools for cluster visualization. The most common techniques include scatter plots, dendrograms, and silhouette plots. By leveraging these techniques, data scientists can generate intuitive insights and determine the quality of their clusters with greater confidence.
Common Techniques for Cluster Visualization
- Principal Component Analysis (PCA) – A dimensionality reduction method that can be used to project high-dimensional cluster data into a lower-dimensional space, making it easier to visualize in 2D or 3D plots.
- t-SNE (t-Distributed Stochastic Neighbor Embedding) – Another dimensionality reduction method that is particularly effective for visualizing high-dimensional data by preserving local similarities.
- Heatmaps – A matrix-based visualization, often used to display similarity or dissimilarity between clusters, particularly when dealing with large datasets.
R Packages for Visualizing Clusters
- ggplot2 – A versatile plotting system that can be used to visualize cluster assignments in scatter plots or other graph types.
- cluster – A package that provides tools for visualizing hierarchical clustering results and silhouette plots.
- factoextra – A package designed specifically for clustering analysis, providing functions for visualizing cluster quality and the cluster’s geometric properties.
- plotly – For interactive 3D plots, especially when using dimensionality reduction methods like PCA or t-SNE.
Key Visualization Techniques in R
Technique | Description | Best Use Case |
---|---|---|
PCA | Reduces dimensions of the data for easier visualization in 2D/3D space. | For understanding global patterns in the data when clusters are high-dimensional. |
t-SNE | Preserves local similarities, useful for separating complex clusters in high-dimensional spaces. | Effective when clusters are not well-separated and difficult to visualize with PCA. |
Heatmaps | Visualizes cluster similarity using color gradients. | Ideal for matrix-based data where you need to highlight differences or similarities across clusters. |
Tip: Always consider the nature of your data when choosing a visualization method. For example, PCA and t-SNE are great for high-dimensional data, while heatmaps are better suited for smaller, matrix-like data sets.
Interpreting Segmentation Results: Assigning Meaning to Your Groups
After performing a segmentation analysis, it's crucial to give context to the resulting segments. This process involves interpreting the characteristics of each group to derive actionable insights. By understanding the distinct traits of each segment, you can tailor strategies that resonate with specific customer needs, behaviors, and preferences. The goal is to extract meaningful patterns that inform decision-making and lead to more effective marketing or product development initiatives.
To begin assigning meaning to segments, you must examine the defining variables that separate them. Common variables in segmentation include demographics, psychographics, purchasing behavior, and more. Once you've identified these factors, you can map them to business objectives, ensuring that each segment aligns with a specific marketing or product strategy.
Steps for Interpreting Your Segments
- Review Key Characteristics: Analyze the most distinguishing features of each segment based on the data. Look for clusters with significant differences in behavior or attributes.
- Label Your Segments: Assign names or labels to each group based on their defining traits. For example, if a segment is mostly young, tech-savvy individuals, consider labeling it "Tech Enthusiasts."
- Assess Alignment with Business Goals: Evaluate how well each segment aligns with your marketing objectives. Does it represent a high-value audience? Is there potential for growth?
The purpose of segmentation is not just to group individuals based on similar traits, but to understand how these groups will behave in real-world scenarios, and how businesses can leverage these insights for strategic advantage.
Example: Segment Breakdown
Segment | Characteristics | Potential Strategy |
---|---|---|
Tech Enthusiasts | Young, highly engaged with technology, frequent online shoppers | Develop targeted ads for new tech products, offer exclusive deals |
Budget-Conscious Shoppers | Price-sensitive, prefers discounts and deals, values practical products | Highlight sales and discount offers, emphasize value for money |
Loyal Customers | Long-term customers, frequent buyers, trust in brand | Offer loyalty rewards, exclusive offers for repeat purchases |
By categorizing and labeling your segments, you not only gain insights into their behavior but also create actionable strategies for engagement. Proper interpretation of segmentation results ensures that your marketing efforts are aligned with the needs and preferences of each group, maximizing the effectiveness of your initiatives.