Automation Using R
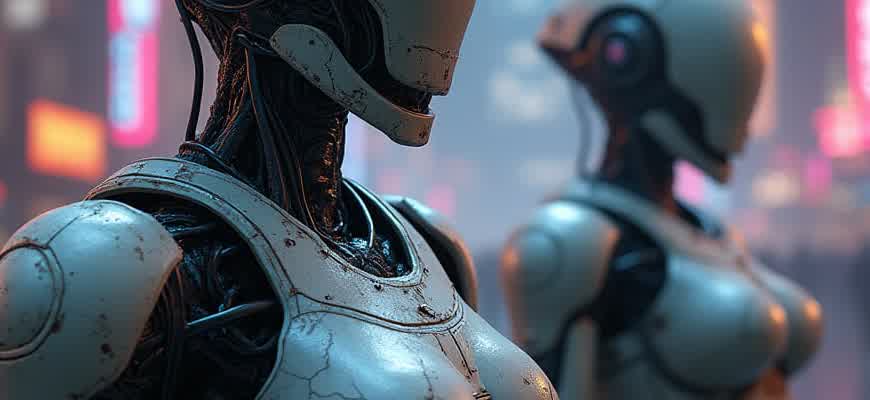
R provides a robust set of tools for automating repetitive data operations, significantly improving productivity and reducing manual errors. It is especially useful in data preprocessing, reporting, and statistical modeling tasks, where consistency and speed are critical. Automation can be achieved through scripting, scheduling, and integrating with external tools.
- Batch processing of large datasets
- Automated generation of reports in PDF, HTML, or Word formats
- Scheduled execution of scripts using cron jobs or Windows Task Scheduler
Automating routine tasks in R not only minimizes human error but also ensures reproducibility and scalability in data projects.
To implement automation in R, a typical setup might involve a combination of packages and system-level tools. The table below shows key components used for different types of tasks:
Task | Tool or Package |
---|---|
Data import/export | readr , data.table |
Report creation | rmarkdown , knitr |
Scheduling | Cron, Task Scheduler, taskscheduleR |
- Write and test your R script
- Use R packages to format outputs
- Schedule the script to run automatically
Automating Recurring Data Workflows with R and Cron Jobs
Automating data processing tasks in R can be efficiently managed by integrating R scripts with Unix-based schedulers like Cron. This approach is ideal for handling regular operations such as ETL (Extract, Transform, Load), API data pulls, and report generation, ensuring consistency and saving time. By writing R scripts for specific tasks and scheduling them with Cron, users can execute processes at predefined intervals without manual intervention.
To enable this setup, a combination of R's scripting capabilities and Cron’s scheduling syntax is used. The R script must be executable and should include all required library calls, authentication details, and file paths. Cron then uses a simple syntax to define the frequency of execution, from every minute to specific days of the week or month.
Implementation Guide
- Ensure Rscript is installed and accessible from the command line.
- Prepare your R script with complete paths and error handling.
- Make the script executable using: chmod +x script.R
- Edit the crontab file using: crontab -e
- Add a Cron entry with the correct schedule and Rscript command.
Note: Use absolute paths in both your R script and Cron job entry to avoid environment-related errors.
- Write a script: /home/user/scripts/daily_etl.R
- Add to crontab: 0 6 * * * /usr/bin/Rscript /home/user/scripts/daily_etl.R
- Check logs regularly for output or errors.
Cron Syntax | Description |
---|---|
0 * * * * | Every hour at minute 0 |
30 2 * * * | At 2:30 AM daily |
0 9 * * 1 | Every Monday at 9:00 AM |
Automating Excel Report Creation with R and the openxlsx Package
Generating structured and formatted Excel reports directly from R can significantly reduce manual workload and minimize the risk of human error. By utilizing the openxlsx package, users can create fully customized Excel files without relying on Microsoft Excel itself or requiring Java-based dependencies.
This tool enables precise control over worksheet content, styling, data validation, and formulas, making it ideal for reproducible reporting workflows. It supports dynamic content generation, allowing integration of analytical outputs into professionally formatted spreadsheets.
Core Workflow for Automated Report Production
- Prepare the data frame with relevant content and calculations.
- Create a new workbook object and add worksheets.
- Write data to sheets using
writeData()
orwriteDataTable()
. - Apply formatting with
createStyle()
andaddStyle()
. - Insert formulas and data validation rules.
- Save the final report with
saveWorkbook()
.
Note: The openxlsx package operates without requiring Excel installation, making it suitable for server-side automation and containerized environments.
- Supports cell styling, merged cells, and conditional formatting.
- Allows insertion of images, charts, and named ranges.
- Enables control over worksheet visibility and protection.
Feature | Function |
---|---|
Add Sheet | addWorksheet() |
Write Data | writeData() |
Create Style | createStyle() |
Save File | saveWorkbook() |
Automated Report Delivery with R and the blastula Package
Generating periodic reports is a common task in data workflows. Automating the delivery of these reports via email can significantly reduce manual effort and ensure consistent communication. The blastula package in R provides a powerful toolkit to craft and send well-formatted HTML emails containing tables, charts, and narrative summaries.
With blastula, users can compose dynamic email bodies using R Markdown, embed R-generated outputs, and schedule messages to be sent through SMTP servers. This allows for fully automated report delivery without leaving the R environment.
Core Steps to Implement Scheduled Email Reports
- Create the report content as an R Markdown document.
- Render the document into an email body using
blastula::render_email()
. - Use
blastula::smtp_send()
to send the email via a configured SMTP server. - Schedule the script with
cronR
or another task scheduler for recurring reports.
Note: Make sure to store sensitive credentials like SMTP passwords using environment variables or encrypted keyrings.
Example of dynamic content inclusion in an email body:
- Custom greetings and timestamps
- Summarized statistics from a data frame
- Inline plots and charts from
ggplot2
Example summary table included in an email:
Metric | Value |
---|---|
Total Sales | $45,000 |
New Customers | 120 |
Churn Rate | 5.6% |
Extracting and Refreshing Online Information with rvest
For automating data extraction from dynamic web resources, the rvest package in R enables users to access structured information from HTML content. It provides tools to target specific nodes using CSS selectors or XPath, which allows consistent parsing of web pages. This is particularly useful for financial analysts, market researchers, and data journalists who need current data points from online tables, price feeds, or news lists.
To maintain data relevance, scheduling scripts that periodically re-run extraction routines is essential. By combining rvest with R’s task scheduling utilities or cron jobs, datasets can be updated on a daily or hourly basis without manual intervention. This is crucial for scenarios where pricing, availability, or other attributes change frequently.
Steps to Implement Scheduled Data Refresh
- Identify static URL structures and page elements to be scraped.
- Write a function using
rvest::read_html()
andhtml_nodes()
to extract needed fields. - Integrate
Sys.sleep()
for ethical scraping with delays between requests. - Use
write.csv()
ordbWriteTable()
to store the outputs. - Configure
taskscheduleR
or system cron to run the script periodically.
Note: Always check the website's robots.txt and terms of service before deploying automated scrapers.
Tool | Purpose | Example |
---|---|---|
rvest::read_html | Load web page HTML | read_html("https://example.com") |
html_nodes | Target elements | html_nodes(".price-tag") |
taskscheduleR | Set automation tasks | taskscheduler_create(...) |
- Supports both XPath and CSS selectors
- Can be used with headless browsers for JS-heavy sites
- Allows combining with dplyr or data.table for fast post-processing
Automating Repetitive CSV File Operations in R
When working with multiple CSV datasets that share a similar structure, manual processing becomes inefficient. R offers a practical solution by automating repetitive file operations such as reading, transforming, and exporting data through loop constructs and functions like list.files() and lapply(). This allows analysts to handle dozens or even hundreds of files with minimal code repetition.
By placing all target CSVs in a designated folder and applying the same transformation to each, users can automate workflows such as column renaming, filtering, and summarization. Output files can then be written to disk systematically with write.csv().
Step-by-Step Execution Using R
- Store all CSVs in a single directory (e.g., ./data/).
- Use list.files() with pattern matching to identify target files.
- Apply lapply() or purrr::map() to perform identical operations.
- Export results to a different directory to keep processed data separate.
Tip: Always check for encoding issues and header consistency before batch processing to avoid transformation errors.
Function | Purpose |
---|---|
list.files() | Collects filenames in the directory |
read.csv() | Reads individual CSV files |
lapply() | Applies a function over a list of items |
write.csv() | Exports data to a new CSV file |
- Ensure uniform column names across input files
- Validate outputs after each transformation stage
- Use dynamic filenames to prevent overwriting files
Building Live-Updating Visual Interfaces with R and Shiny
Interactive data platforms built with R and Shiny enable real-time insights by connecting directly to live data sources. These platforms refresh visualizations dynamically, eliminating the need for manual updates. This is especially useful for tracking KPIs, monitoring system performance, or following market trends.
The application reacts to user input, server-side calculations, or automated time-based triggers. By using reactive expressions and observers, R developers can build a responsive interface that reflects the latest available data, integrating inputs from APIs, databases, or sensor feeds.
Key Elements of a Responsive Dashboard
- Reactive data sources – Pulls from SQL, CSV, or APIs without needing reloads.
- User-driven controls – Filters, selectors, and input forms for personalized views.
- Live plots and tables – Powered by plotly, ggplot2, or DT packages.
Real-time dashboards built in Shiny automatically adapt to new data, providing continuous visibility without developer intervention.
- Define a reactive expression that fetches and transforms new data.
- Link this expression to UI elements such as charts or tables.
- Use invalidateLater() to set timed refresh intervals.
Component | Function | Package |
---|---|---|
Live Data Table | Displays real-time records | DT |
Dynamic Plot | Visualizes trends as they evolve | plotly |
Input Filter | Customizes view for the user | shinyWidgets |
Automating Database Maintenance with R Scripts
R is widely used for various data-related tasks, including the automation of routine database maintenance. Through the use of scripts, R can help automate database backup, cleanup, and performance optimization tasks. By writing efficient R code, users can save time and ensure that database operations are performed consistently without manual intervention.
R scripts can be scheduled to run at specific times or triggered by certain events, making them a valuable tool for database administrators. This approach not only reduces human error but also streamlines the maintenance process by automating repetitive tasks such as data validation, error logging, and database optimization.
Common Database Maintenance Tasks Automated Using R
- Backup Management: Automating regular backups of databases to ensure data safety.
- Data Cleanup: Writing scripts to identify and remove redundant or outdated records from the database.
- Performance Tuning: Scheduling scripts that monitor and optimize database performance, such as indexing or query optimization.
Example of Automating a Backup Process
Here’s an example of an R script that can be used to automate the process of database backup:
# Example of an R script to back up a database library(DBI) con <- dbConnect(RMySQL::MySQL(), dbname = "mydb", host = "localhost", user = "root", password = "password") backup_file <- paste("backup_", Sys.Date(), ".sql", sep = "") dbWriteTable(con, name = "backup", value = "SELECT * FROM my_table;", file = backup_file) dbDisconnect(con)
Key Points to Remember
Automating tasks with R ensures that database maintenance is done consistently and accurately.
Scheduling R scripts can reduce the workload of database administrators by performing critical tasks at predefined intervals.
Advantages of Using R for Automation
Advantage | Description |
---|---|
Flexibility | R offers a wide variety of packages for database connections and operations, making it highly customizable for different tasks. |
Reusability | R scripts can be reused for multiple databases or across different projects, ensuring efficiency and reducing redundancy. |
Scalability | As databases grow, R scripts can be easily modified to handle larger datasets or more complex operations. |
Automating R Workflows with GitHub Actions
GitHub Actions allows the automation of various tasks, including running R scripts and managing workflows for R-based projects. By integrating R into GitHub's continuous integration (CI) platform, developers can automate the testing, deployment, and execution of R code in response to specific events or changes in the repository. This approach streamlines processes and ensures consistency across different environments.
Triggering R workflows through GitHub Actions is an efficient method for automating the execution of scripts and analysis pipelines. It enables teams to run automated tests, deploy models, or update reports every time there is a commit or pull request. By setting up triggers within GitHub Actions, users can ensure that their R-based projects are always up-to-date and functional.
Setting Up R Workflows with GitHub Actions
To get started with triggering R workflows, you need to configure GitHub Actions in your repository. Below is a step-by-step guide:
- Create a new file in your repository under the .github/workflows directory, such as r-workflow.yml.
- Define the trigger event. For example, you can set it to run when there is a push or pull request to a specific branch.
- Specify the jobs to execute within the workflow, such as running R scripts, installing dependencies, and testing the code.
- Use actions like set up-r to install R and actions/setup-Renv to set up R environments.
Important: Make sure that your R environment is properly set up in the action to ensure compatibility with the version used in your scripts.
Example Workflow Configuration
The following is an example configuration for an R workflow in GitHub Actions:
name: Run R Scripts on Push on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout repository uses: actions/checkout@v2 - name: Set up R uses: r-lib/actions/setup-r@v2 - name: Install dependencies run: | install.packages("devtools") devtools::install_github("tidyverse/ggplot2") - name: Run tests run: Rscript -e "testthat::test_dir('tests')"
This configuration will run every time there is a push to the main branch and execute the necessary tasks such as installing R dependencies and running tests.
Key Benefits of Automation with GitHub Actions
- Consistency: Automating the process ensures that R scripts are executed in a consistent environment every time.
- Speed: GitHub Actions accelerates tasks like testing and deployment by automating repetitive steps.
- Integration: Easy integration with other GitHub tools and workflows helps create a seamless CI/CD pipeline for R projects.
Common Use Cases for R Workflows
Use Case | Description |
---|---|
Automated Testing | Trigger tests to validate R scripts whenever changes are made to the repository. |
Model Deployment | Run models and deploy them automatically after successful tests or commits. |
Report Generation | Automatically generate and update reports when the data or analysis scripts are changed. |