Java List Segmentation
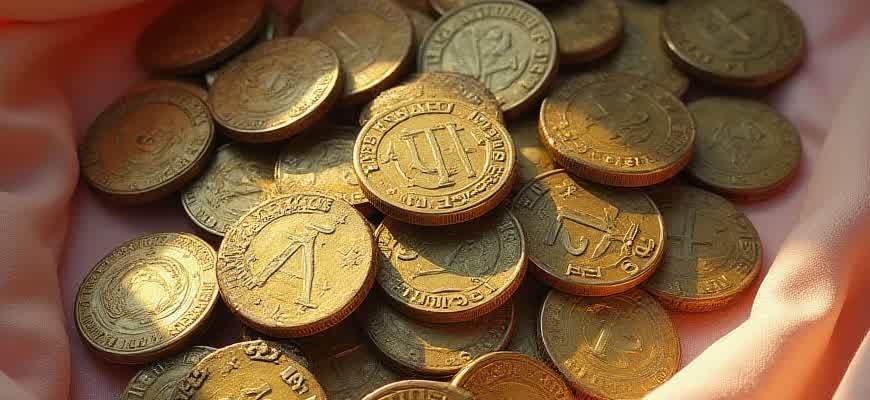
In Java, the process of dividing a list into smaller, more manageable parts is often referred to as "list segmentation." This technique is useful when working with large datasets or when a specific operation needs to be performed on smaller portions of a list.
There are different methods for segmenting lists, depending on the desired outcome. One of the most common approaches is to split the list into equal-sized chunks. The following methods are frequently used in Java:
- Using Sublist Method: Java's
List.subList()
method provides an easy way to retrieve a sublist from an existing list, which can be considered a form of segmentation. - Custom Split Logic: In some cases, developers implement custom logic to segment the list based on specific criteria or conditions.
- Using Streams: Java Streams provide a functional approach to splitting and processing list data in parallel or sequential chunks.
Important: Always ensure that the indices are valid when usingsubList()
to avoidIndexOutOfBoundsException
.
For example, consider the following table of different segmentation strategies:
Method | Description | Use Case |
---|---|---|
Sublist | Extracts a portion of the list by specifying start and end indices. | When a simple chunk of data is needed from a large list. |
Custom Split | Divides a list based on custom logic or business rules. | When you need more control over how the list is segmented. |
Streams | Uses streams to partition the list into chunks for parallel processing. | For handling large datasets efficiently in parallel operations. |
Dividing Large Lists into Smaller Chunks in Java
In Java, working with large datasets often requires breaking them down into smaller, manageable chunks. This helps improve memory usage, processing time, and general performance. Dividing large lists into smaller pieces can be useful when handling batch processing, pagination, or managing data that exceeds the available memory.
There are several techniques you can use to segment a list. Each approach offers different trade-offs in terms of code simplicity, efficiency, and scalability. Below are some common methods for splitting large lists into smaller parts.
Using SubList Method
The subList() method from the List interface is a convenient way to divide a large list into smaller sublists. It takes two arguments: the starting index and the ending index, and returns a view of the list within that range.
Note: The subList()
method does not create a new list. It provides a view of the original list, so modifications to the sublist will affect the original list.
- First, calculate the required chunk size.
- Then, loop through the list, creating sublists using
subList()
based on the calculated indices. - Finally, store each sublist in a new list or process it as needed.
Example Code
ListlargeList = new ArrayList<>(); // Add elements to the list int chunkSize = 100; for (int i = 0; i < largeList.size(); i += chunkSize) { List chunk = largeList.subList(i, Math.min(i + chunkSize, largeList.size())); // Process the chunk }
Using Custom Chunking Logic
For more flexibility, you can manually create smaller lists by iterating over the original list and adding elements to new sublists. This is a good option if you need more control over the chunk creation process, such as ensuring that the chunks meet specific conditions.
- Initialize a new empty list for each chunk.
- Iterate over the original list, adding elements to the current chunk.
- Once a chunk reaches the desired size, add it to a list of chunks and create a new chunk.
Example Code
ListlargeList = new ArrayList<>(); List > chunkedLists = new ArrayList<>(); int chunkSize = 100; List
currentChunk = new ArrayList<>(); for (Integer value : largeList) { currentChunk.add(value); if (currentChunk.size() == chunkSize) { chunkedLists.add(new ArrayList<>(currentChunk)); currentChunk.clear(); } } if (!currentChunk.isEmpty()) { chunkedLists.add(currentChunk); // Add any remaining elements as the last chunk }
Using Streams for Chunking
Another modern approach involves using Java Streams for chunking. This can be especially effective when working with large lists in a functional programming style. Below is a method for splitting a list using Stream
and Collectors.groupingBy
.
Method | Description |
---|---|
subList() | Returns a view of a portion of the list between the specified indices. |
Manual Chunking | Manually splits the list into chunks using iteration and a condition to create new sublists. |
Streams | Uses Java Streams API to group elements based on a defined condition. |
Implementing Custom Segmentation Logic for Java Lists
When working with Java lists, there are various scenarios where you might need to split or group elements based on specific criteria. A custom segmentation approach allows you to tailor how the list is divided, whether it’s by range, type, or a more complex condition. Implementing such logic often involves iterating through the list, evaluating each element, and creating sublists accordingly. This process is crucial when dealing with large datasets where you need to optimize performance or improve clarity by working with smaller chunks of data.
To implement custom segmentation, you can define rules for splitting the list based on specific characteristics or conditions. You could use conditions like value ranges, object types, or custom thresholds. The approach might involve using built-in Java methods or crafting your own algorithms to achieve the desired grouping behavior.
Basic Approach
One of the most common ways to achieve list segmentation is by iterating through the original list and adding elements to new sublists based on specific conditions. Below is a simple example of this process:
- Define the condition for segmentation (e.g., a threshold value, object properties).
- Iterate over the list and evaluate each element against the condition.
- Create a new sublist for each group based on the evaluation result.
- Repeat until the entire list has been processed.
Example: Segmentation by Value Range
Consider a list of integers that you want to segment into sublists based on predefined ranges:
Range | Condition | Sublist |
---|---|---|
0-10 | Value <= 10 | [1, 2, 5, 9] |
11-20 | Value > 10 && Value <= 20 | [15, 18] |
21+ | Value > 20 | [25, 30, 40] |
Custom segmentation logic can help break large data sets into manageable chunks, improving both readability and performance when processing elements sequentially.
Advanced Segmentation with Custom Conditions
For more advanced segmentation, you might need to define more complex logic based on properties or computed values. This could involve applying predicates, using lambda expressions, or working with Java Streams. Such methods provide greater flexibility in how the list is divided and allow for more sophisticated grouping behavior. The key is to ensure that your conditions are both efficient and scalable, especially when handling large collections.
Optimizing Memory Usage with List Segmentation Techniques
In large-scale applications where memory efficiency is critical, managing collections of data becomes a major concern. One effective approach to optimizing memory usage is through segmenting large lists into smaller, more manageable parts. This technique ensures that memory is allocated only when necessary, improving both performance and resource utilization. Segmentation helps to avoid the overhead of storing excessive data in memory at once, enabling better control over memory consumption.
Segmenting lists also allows developers to process data in chunks, thus minimizing the risk of memory-related issues such as OutOfMemoryErrors. By dividing large datasets into smaller, logical segments, you can optimize garbage collection, improve cache performance, and reduce the time it takes to load or process the entire list. This is particularly useful in environments with limited memory resources, such as mobile applications or embedded systems.
Key Techniques for Effective List Segmentation
- Fixed-size Chunking: Dividing lists into fixed-size sublists can make data management predictable and easy to implement.
- Lazy Loading: Segments of the list are loaded on demand, ensuring only the necessary data resides in memory at any given time.
- Sliding Windows: A sliding window approach can help process segments of data sequentially without reloading or holding unnecessary parts of the list.
Advantages of List Segmentation
- Reduced Memory Footprint: By processing data in smaller parts, you minimize memory consumption.
- Improved Performance: Smaller segments result in quicker data retrieval and processing times.
- Efficient Garbage Collection: Less memory retention means that unused segments can be collected more effectively by the garbage collector.
Tip: When segmenting a list, it's essential to ensure that the segmentation method aligns with the application's data access patterns to avoid unnecessary performance hits.
Example of List Segmentation
Segment Type | Description |
---|---|
Fixed-size Chunk | Divides the list into sublists of equal size, making the process simple and efficient. |
Lazy Loading | Loads data into memory only when required, minimizing unnecessary resource usage. |
Sliding Window | Processes overlapping segments of the list, useful for streaming or real-time processing. |
Handling Lists with Uneven Distribution in Java
When dealing with lists in Java, uneven distribution of elements can lead to challenges during segmentation. This issue typically arises when the number of elements is not evenly divisible by the desired segment size. In such cases, it is important to implement an approach that ensures all elements are processed, even if the last segment contains fewer items. Understanding how to manage such situations will improve the efficiency and flexibility of your data handling operations.
To effectively manage lists with uneven distribution, several strategies can be employed. One common solution is to dynamically adjust the segment size based on the number of elements in the list. Below are a few approaches to handle such cases in Java:
Strategies for Handling Uneven Lists
- Use of SubLists: One of the most straightforward methods is utilizing the
subList()
method, which allows you to create smaller lists from the main list. You can loop through the list and extract sublists of a predefined size. - Custom Partition Logic: Implementing a custom loop to handle uneven partitions allows more control over how to split the list. In this case, a check can be added to process the remaining elements when the segment size is not a perfect divisor of the total number of elements.
- Padding the List: Another approach is to add dummy elements to the list to ensure an even distribution. This is especially useful when working with systems that require equal-sized partitions.
Example of Custom Segmentation
Listnumbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7); int segmentSize = 3; List > segments = new ArrayList<>(); for (int i = 0; i < numbers.size(); i += segmentSize) { segments.add(numbers.subList(i, Math.min(i + segmentSize, numbers.size()))); }
When implementing custom partitioning, it is important to ensure that the last segment can accommodate the remaining items, which may not fully fill the segment size.
Considerations for Uneven Lists
While segmenting a list, consider the following:
- Performance: The efficiency of segmenting a large list depends on the chosen method. Using
subList()
is generally efficient, but creating multiple new lists can have overhead in terms of memory usage. - Boundary Handling: Make sure the logic accounts for the last segment correctly, especially when it contains fewer elements than the other segments.
- Use Case: The chosen method should align with the use case. For example, padding might be appropriate when processing fixed-size blocks, but dynamic partitioning works best for variable-length data.
Comparison of Methods
Method | Pros | Cons |
---|---|---|
subList() | Simple, easy to implement | Potential memory overhead with large lists |
Custom Logic | Flexible, fully customizable | More complex to implement |
Padding | Ensures equal-size segments | Can introduce unnecessary elements |
Using Java Streams for Advanced List Segmentation
Java Streams offer a powerful way to process collections of data, enabling efficient and clean segmentation of lists based on various criteria. Instead of relying on manual iteration and conditional checks, Streams allow for a more declarative approach to dividing a list into smaller, manageable segments. This technique is especially useful when working with large datasets or when complex filtering and grouping operations are required.
To achieve advanced list segmentation, developers can leverage different stream operations such as filtering, mapping, and collecting. These operations can be combined to split the data into sublists based on custom criteria, making the process both efficient and readable. Below, we explore some of the most effective strategies for implementing this in Java.
Segmentation using `Collectors.groupingBy`
One of the most common ways to segment a list in Java using Streams is through the groupingBy collector. This operation allows you to classify elements of a stream into groups based on a specific criterion.
- For example, grouping elements by their length or by some attribute of the objects in the list.
- It can also be used to segment data into sublists based on values such as date, category, or type.
Important: The groupingBy method returns a map where each key represents a group, and each value is a list of items that share that key’s criterion.
The following example demonstrates how to use groupingBy
to segment a list of strings by their length:
List words = Arrays.asList("apple", "banana", "cherry", "date", "fig", "grape");
Map> groupedByLength = words.stream()
.collect(Collectors.groupingBy(String::length));
groupedByLength.forEach((length, wordList) ->
System.out.println("Length " + length + ": " + wordList));
Advanced Segmentation with `Collectors.partitioningBy`
If you need to divide your list into two segments based on a predicate, you can use the partitioningBy collector. This approach is ideal when you want to categorize data into two distinct groups, such as "passed" vs. "failed" or "even" vs. "odd".
- The
partitioningBy
collector divides the stream into two categories. - The result is a map with two keys:
true
andfalse
, representing the outcome of the predicate.
Note: The partitioningBy method is ideal for situations where only two groups are required. If more than two groups are needed, consider using groupingBy
.
Here is an example of partitioning a list of numbers into even and odd values:
List numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
Map> partitioned = numbers.stream()
.collect(Collectors.partitioningBy(n -> n % 2 == 0));
partitioned.forEach((isEven, list) ->
System.out.println((isEven ? "Even" : "Odd") + ": " + list));
Table of Common List Segmentation Methods
Method | Usage | Result |
---|---|---|
groupingBy |
Segments based on a classification criterion | Map where key is the criterion, and value is the list of matching elements |
partitioningBy |
Partitions based on a boolean condition | Map with two entries: true and false |
Collectors.toList |
Collects elements into a list | A list containing the elements processed by the stream |